Êíèãà: C# 2008 Programmer
Wiring All the Event Handlers
Wiring All the Event Handlers
With the helper functions defined, let's wire up all the event handlers for the various controls. First, code the Form1_Load
event handler as follows:
private void Form1_Load(object sender, EventArgs e) {
//---find out the app's path---
appPath = Path.GetDirectoryName(
System.Reflection.Assembly.GetExecutingAssembly().GetName().CodeBase);
//---set the feed list to be stored in the app's folder---
feedsList = appPath + feedsList;
try {
//---create the root node---
TreeNode node = new TreeNode() {
ImageIndex = ICO_CLOSE,
SelectedImageIndex = ICO_OPEN,
Text = "Subscribed Feeds"
};
//---add the node to the tree---
TreeView1.Nodes.Add(node);
TreeView1.SelectedNode = node;
} catch (Exception ex) {
MessageBox.Show(ex.Message);
return;
}
try {
//---load all subscribed feeds---
if (File.Exists(feedsList)) {
TextReader textreader = File.OpenText(feedsList);
//---read URLs of all the subscribed feeds---
string[] feeds = textreader.ReadToEnd().Split('|');
textreader.Close();
//---add all the feeds to the tree---
for (int i = 0; i <= feeds.Length - 2; i++)
SubscribeFeed(feeds[i]);
} else {
//---pre-subscribe to a few feed(s)---
SubscribeFeed(
"http://www.wrox.com/WileyCDA/feed/RSS_WROX_ALLNEW.xml");
}
} catch (Exception ex) {
MessageBox.Show(ex.Message);
}
}
When the form is first loaded, you have to create a root node for the TreeView
control and load all the existing feeds. All subscribed feeds are saved in a plain text file (Feeds.txt
), in the following format:
Feed URL|Feed URL|Feed URL|
An example is:
http://news.google.com/?output=rss|http://rss.cnn.com/rss/cnn_topstories.rss|
If there are no existing feeds (that is, if Feeds.txt
does not exist), subscribe to at least one feed.
In the Click
event handler of the Subscribe MenuItem
control, prompt the user to input a feed's URL, and then subscribe to the feed. If the subscription is successful, save the feed URL to file:
private void mnuSubscribe_Click(object sender, EventArgs e) {
if (!IsConnected()) {
MessageBox.Show("You are not connected to the Internet.");
return;
}
//---add a reference to Microsoft.VisualBasic.dll---
string URL = Microsoft.VisualBasic.Interaction.InputBox(
"Please enter the feed URL", "Feed URL", lastURLEntered, 0, 0);
if (URL != String.Empty) {
lastURLEntered = URL;
//---if feed is subscribed successfully---
if (SubscribeFeed(URL)) {
//---save in feed list---
TextWriter textwriter = File.AppendText(feedsList);
textwriter.Write(URL + "|");
textwriter.Close();
} else {
MessageBox.Show("Feed not subscribed. " +
"Please check that you have entered " +
"the correct URL and that you have " +
"Internet access.");
}
}
}
C# does not include the InputBox()
function that is available in VB.NET to get user's input (see Figure 18-14). Hence, it is a good idea to add a reference to the Microsoft.VisualBasic.dll
library and use it as shown in the preceding code.
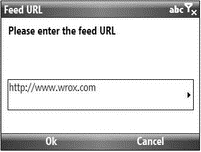
Figure 18-14
Whenever a node in the TreeView
control is selected, you should perform a check to see if it is a posting node and enable/disable the MenuItem controls appropriately (see Figure 18-15):
//---fired after a node in the TreeView control is selected---
private void TreeView1_AfterSelect(object sender, TreeViewEventArgs e) {
//---if a feed node is selected---
if (e.Node.ImageIndex != ICO_POST && e.Node.Parent != null) {
mnuUnsubscribe.Enabled = true;
mnuRefreshFeed.Enabled = true;
} else {
//---if a post node is selected---
mnuUnsubscribe.Enabled = false;
mnuRefreshFeed.Enabled = false;
}
}
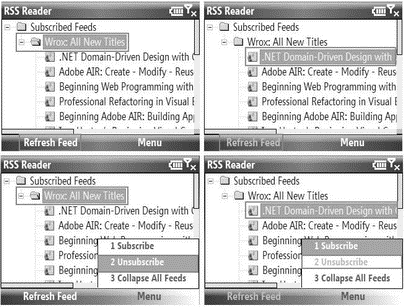
Figure 18-15
When the user selects a post using the Select button on the navigation pad, Form2
containing the WebBrowser
control is loaded and its content set accordingly (see Figure 18-16). This is handled by the KeyDown
event handler of the TreeView control:
//---fired when a node in the TreeView is selected
// and the Enter key pressed---
private void TreeView1_KeyDown(object sender, KeyEventArgs e) {
TreeNode node = TreeView1.SelectedNode;
//---if the Enter key was pressed---
if (e.KeyCode == System.Windows.Forms.Keys.Enter) {
//---if this is a post node---
if (node.ImageIndex == ICO_POST) {
//---set the title of Form2 to title of post---
frm2.Text = node.Text;
//---modifier for webBrowser1 in Form2 must be set to
// Internal---
//---set the webbrowser control to display the post content---
frm2.webBrowser1.DocumentText = node.Tag.ToString();
//---show Form2---
frm2.Show();
}
}
}
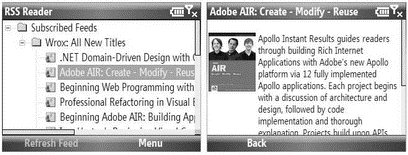
Figure 18-16
To unsubscribe a feed, you remove the feed's URL from the text file and then remove the feed node from the TreeView
control. This is handled by the Unsubscribe MenuItem
control:
//---Unsubscribe a feed---
private void mnuUnsubscribe_Click(object sender, EventArgs e) {
//---get the node to unsubscribe---
TreeNode CurrentSelectedNode = TreeView1.SelectedNode;
//---confirm the deletion with the user---
DialogResult result =
MessageBox.Show("Remove " + CurrentSelectedNode.Text + "?",
"Unsubscribe", MessageBoxButtons.YesNo,
MessageBoxIcon.Question, MessageBoxDefaultButton.Button1);
try {
if (result == DialogResult.Yes) {
//---URL To unsubscribe---
string urlToUnsubscribe = CurrentSelectedNode.Tag.ToString();
//---load all the feeds from feeds list---
TextReader textreader = File.OpenText(feedsList);
string[] feeds = textreader.ReadToEnd().Split('|');
textreader.Close();
//---rewrite the feeds list omitting the one to be
// unsubscribed---
TextWriter textwriter = File.CreateText(feedsList);
for (int i = 0; i <= feeds.Length - 2; i++) {
if (feeds[i] != urlToUnsubscribe) {
textwriter.Write(feeds[i] + "|");
}
}
textwriter.Close();
//---remove the node from the TreeView control---
CurrentSelectedNode.Remove();
MessageBox.Show("Feed unsubscribed!");
}
} catch (Exception ex) {
MessageBox.Show(ex.Message);
}
}
When the user needs to refresh a feed, first make a backup copy of the feed XML document and proceed to subscribe to the same feed again. If the subscription is successful, remove the node containing the old feed. If the subscription is not successful (for example, when a device is disconnected from the Internet), restore the backup feed XML document. This is handled by the Refresh Feed MenuItem
control:
//---refresh the current feed---
private void mnuRefreshFeed_Click(object sender, EventArgs e) {
//---if no Internet connectivity---
if (!IsConnected()) {
MessageBox.Show("You are not connected to the Internet."); return;
}
//---get the node to be refreshed---
TreeNode CurrentSelectedNode = TreeView1.SelectedNode;
string url = CurrentSelectedNode.Tag.ToString();
//---get the filename of the feed---
string FileName =
appPath + @"" + RemoveSpecialChars(url) + ".xml";
try {
//---make a backup copy of the current feed---
File.Copy(FileName, FileName + "_Copy", true);
//---delete feed from local storage---
File.Delete(FileName);
//---load the same feed again---
if (SubscribeFeed(url)) {
//---remove the node to be refreshed---
CurrentSelectedNode.Remove();
} else //---the subscription(refresh) failed---
{
//---restore the deleted feed file---
File.Copy(FileName + "_Copy", FileName, true);
MessageBox.Show("Refresh not successful. Please try again.");
}
//---delete the backup file---
File.Delete(FileName + "_Copy");
} catch (Exception ex) {
MessageBox.Show("Refresh failed (" + ex.Message + ")");
}
}
In the Click
event handler for the Collapse All Feeds MenuItem
control, use the CollapseAll()
method from the TreeView
control to collapse all the nodes:
private void mnuCollapseAllFeeds_Click(object sender, EventArgs e) {
TreeView1.CollapseAll();
}
Finally, code the Click
event handler in the Back MenuItem
control in Form2
as follows:
private void mnuBack_Click(object sender, EventArgs e) {
this.Hide();
}
That's it! You are now ready to test the application.
- 4.4.4 The Dispatcher
- EVENT MEMORY SIZE
- About the author
- Chapter 7. The state machine
- Chapter 5 Installing and Configuring VirtualCenter 2.0
- Chapter 13. rc.firewall file
- Appendix E. Other resources and links
- 3.2.1.2. Íà÷àëüíîå âûäåëåíèå ïàìÿòè: malloc()
- Example NAT machine in theory
- The final stage of our NAT machine
- Compiling the user-land applications
- Installation on Red Hat 7.1