Книга: Beginning Android
Spin Control
Spin Control
In Android, the Spinner
is the equivalent of the drop-down selector you might find in other toolkits (e.g., JComboBox
in Java/Swing). Pressing the center button on the D-pad pops up a selection dialog for the user to choose an item from. You basically get the ability to select from a list without taking up all the screen space of a ListView
, at the cost of an extra click or screen tap to make a change.
As with ListView
, you provide the adapter for data and child views via setAdapter()
and hook in a listener object for selections via setOnItemSelectedListener()
.
If you want to tailor the view used when displaying the drop-down perspective, you need to configure the adapter, not the Spinner
widget. Use the setDropDownViewResource()
method to supply the resource ID of the view to use.
For example, culled from the Selection/Spinner
sample project, here is an XML layout for a simple view with a Spinner
:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<TextView
android:id="@+id/selection"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
/>
<Spinner android:id="@+id/spinner"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:drawSelectorOnTop="true"
/>
</LinearLayout>
This is the same view as shown in the previous section, just with a Spinner
instead of a ListView
. The Spinner
property android:drawSelectorOnTop
controls whether the arrows are drawn on the selector button on the right side of the Spinner
UI.
To populate and use the Spinner
, we need some Java code:
public class SpinnerDemo extends Activity
implements AdapterView.OnItemSelectedListener {
TextView selection;
String[] items={"lorem", "ipsum", "dolor", "sit", "amet",
"consectetuer", "adipiscing", "elit", "morbi", "vel",
"ligula", "vitae", "arcu", "aliquet", "mollis",
"etiam", "vel", "erat", "placerat", "ante",
"porttitor", "sodales", "pellentesque", "augue", "purus"};
@Override
public void onCreate(Bundle icicle) {
super.onCreate(icicle);
setContentView(R.layout.main);
selection = (TextView)findViewById(R.id.selection);
Spinner spin = (Spinner)findViewById(R.id.spinner);
spin.setOnItemSelectedListener(this);
ArrayAdapter<String> aa = new ArrayAdapter<String>(this,
android.R.layout.simple_spinner_item, items);
aa.setDropDownViewResource(
android.R.layout.simple_spinner_dropdown_item);
spin.setAdapter(aa);
}
public void onItemSelected(AdapterView<?> parent,
View v, int position, long id) {
selection.setText(items[position]);
}
public void onNothingSelected(AdapterView<?> parent) {
selection.setText("");
}
}
Here, we attach the activity itself as the selection listener (spin.setOnItemSelectedListener(this)
). This works because the activity implements the OnItemSelectedListener
interface. We configure the adapter not only with the list of fake words, but also with a specific resource to use for the drop-down view (via aa.setDropDownViewResource()
). Also note the use of android.R.layout.simple_spinner_item
as the built-in View for showing items in the spinner itself. Finally, we implement the callbacks required by OnItemSelectedListener
to adjust the selection label based on user input.
The resulting application is shown in Figures 8-2 and 8-3.
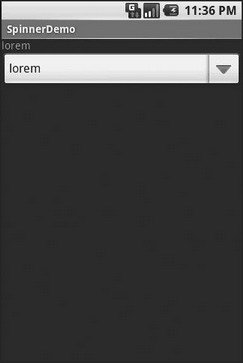
Figure 8-2. The SpinnerDemo sample application, as initially launched
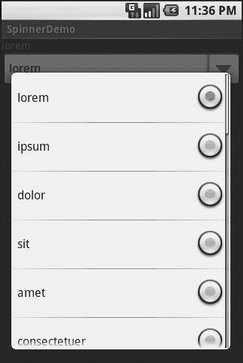
Figure 8-3. The same application, with the spinner drop-down list displayed
- LOCK ACQUIRE SPINS
- Introduction to Microprocessors and Microcontrollers
- Data sending and control session
- Data Binding Using the GridView Control
- Configure Access Control
- Using the kill Command to Control Processes
- 3.4.4. Concurrency Control
- Controlling Services at Boot with Administrative Tools
- Basic Shell Control
- Using Priority Scheduling and Control
- Controlling Apache with Fedora's service Command
- Controlling Apache with Fedora's chkconfig Command