Книга: Beginning Android
Lists of Naughty and Nice
Lists of Naughty and Nice
The classic listbox widget in Android is known as ListView
. Include one of these in your layout, invoke setAdapter()
to supply your data and child views, and attach a listener via setOnItemSelectedListener()
to find out when the selection has changed. With that, you have a fully-functioning listbox.
However, if your activity is dominated by a single list, you might well consider creating your activity as a subclass of ListActivity
, rather than the regular Activity
base class. If your main view is just the list, you do not even need to supply a layout — ListActivity
will construct a full-screen list for you. If you do want to customize the layout, you can, so long as you identify your ListView
as @android:id/list
, so ListActivity
knows which widget is the main list for the activity.
For example, here is a layout pulled from the Selection/List
sample project. This sample along with all others in this chapter can be found in the Source Code area of http://apress.com.
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<TextView
android:id="@+id/selection"
android:layout_width="fill_parent"
android:layout_height="wrap_content"/>
<ListView
android:id="@android:id/list"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:drawSelectorOnTop="false"
/>
</LinearLayout>
It is just a list with a label on top to show the current selection.
The Java code to configure the list and connect the list with the label is:
public class ListViewDemo extends ListActivity {
TextView selection;
String[] items={"lorem", "ipsum", "dolor", "sit", "amet",
"consectetuer", "adipiscing", "elit", "morbi", "vel",
"ligula", "vitae", "arcu", "aliquet", "mollis",
"etiam", "vel", "erat", "placerat", "ante",
"porttitor", "sodales", "pellentesque", "augue", "purus"};
@Override
public void onCreate(Bundle icicle) {
super.onCreate(icicle);
setContentView(R.layout.main);
setListAdapter(new ArrayAdapter<String>(this,
android.R.layout.simple_list_item_1,
items));
selection=(TextView)findViewById(R.id.selection);
}
public void onListItemClick(ListView parent, View v, int position,
long id) {
selection.setText(items[position]);
}
}
With ListActivity
, you can set the list adapter via setListAdapter()
— in this case, providing an ArrayAdapter
wrapping an array of nonsense strings. To find out when the list selection changes, override onListItemClick()
and take appropriate steps based on the supplied child view and position (in this case, updating the label with the text for that position).
The results are shown in Figure 8-1.
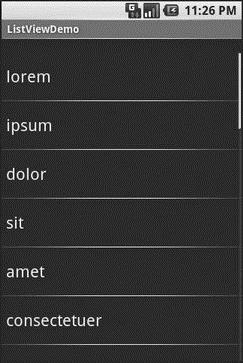
Figure 8-1. The ListViewDemo sample application
- Разработка приложений баз данных InterBase на Borland Delphi
- Open Source Insight and Discussion
- Introduction to Microprocessors and Microcontrollers
- Chapter 6. Traversing of tables and chains
- Chapter 8. Saving and restoring large rule-sets
- Chapter 11. Iptables targets and jumps
- Chapter 5 Installing and Configuring VirtualCenter 2.0
- Chapter 16. Commercial products based on Linux, iptables and netfilter
- Appendix A. Detailed explanations of special commands
- Appendix B. Common problems and questions
- Appendix E. Other resources and links
- IP filtering terms and expressions