Книга: Beginning Android
Just Another Box to Check
Just Another Box to Check
The classic checkbox has two states: checked and unchecked. Clicking the checkbox toggles between those states to indicate a choice (e.g., “Add rush delivery to my order”).
In Android, there is a CheckBox
widget to meet this need. It has TextView
as an ancestor, so you can use TextView
properties like android:textColor
to format the widget.
Within Java, you can invoke:
• isChecked()
to determine if the checkbox has been checked
• setChecked()
to force the checkbox into a checked or unchecked state
• toggle()
to toggle the checkbox as if the user checked it
Also, you can register a listener object (in this case, an instance of OnCheckedChangeListener
) to be notified when the state of the checkbox changes.
For example, from the Basic/CheckBox
project, here is a simple checkbox layout:
<?xml version="1.0" encoding="utf-8"?>
<CheckBox xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/check"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="This checkbox is: unchecked" />
The corresponding CheckBoxDemo.java
retrieves and configures the behavior of the checkbox:
public class CheckBoxDemo extends Activity
implements CompoundButton.OnCheckedChangeListener {
CheckBox cb;
@Override
public void onCreate(Bundle icicle) {
super.onCreate(icicle);
setContentView(R.layout.main);
cb=(CheckBox)findViewById(R.id.check);
cb.setOnCheckedChangeListener(this);
}
public void onCheckedChanged(CompoundButton buttonView,
boolean isChecked) {
if (isChecked) {
cb.setText("This checkbox is: checked");
} else {
cb.setText("This checkbox is: unchecked");
}
}
}
Note that the activity serves as its own listener for checkbox state changes since it implements the OnCheckedChangeListener
interface (via cb.setOnCheckedChangeListener(this)
). The callback for the listener is onCheckedChanged()
, which receives the checkbox whose state has changed and what the new state is. In this case, we update the text of the checkbox to reflect what the actual box contains.
The result? Clicking the checkbox immediately updates its text, as you can see in Figures 6-4 and 6-5.
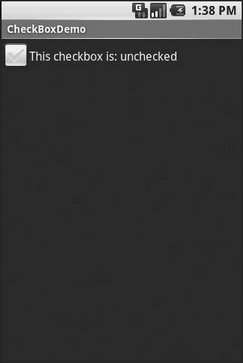
Figure 6-4. The CheckBoxDemo sample application, with the checkbox unchecked
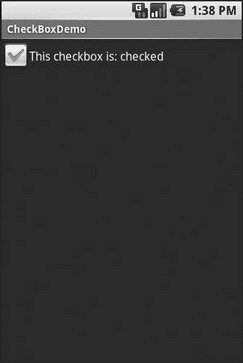
Figure 6-5. The same application, now with the checkbox checked
- Checksum
- Условия CHECK
- It’s All The Same, Just Different
- 12.6.4. Logcheck
- 2.12 Оконные менеджеры «BlackBox» и «FluxBox»
- Adjusting Volume
- Checking for the Availability of the Loopback Interface
- Adding Some Error Checking
- A Predeployment Planning Checklist
- 3.3.4 Spellchecking on the Fly with Flyspell
- Checking Hardware Compatibility
- Chapter 11. BusyBox