Книга: C# 2008 Programmer
Understanding Namespaces and Assemblies
Understanding Namespaces and Assemblies
As you know from Chapter 1, the various class libraries in the .NET Framework are organized using namespaces. So how do namespaces relate to assemblies? To understand the relationship between namespaces and assemblies, it's best to take a look at an example.
Create a new Class Library project in Visual Studio 2008, and name it ClassLibrary1
. In the default Class1.cs
, populate it with the following:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace Learn2develop.net {
public class Class1 {
public void DoSomething() {
}
}
}
Observe that the definition of Class1
is enclosed within the Learn2develop.net
namespace. The class also contains the DoSomething()
method.
Add a new class to the project by right-clicking on the project's name in Solution Explorer and selecting Add?Class (see Figure 15-21).
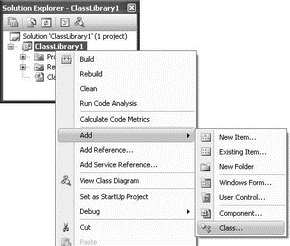
Figure 15-21
Use the default name of Class2.cs
. In the newly added Class2.cs
, code the following:
using System;
is enclosed within the same namespace —
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace Learn2develop.net {
public class Class2 {
public void DoSomething() {
}
}
}
Class2Learn2develop.net
, and it also has a DoSomething()
method. Compile the ClassLibrary1
project so that an assembly is generated in the binDebug folder of the project — ClassLibrary1.dll
. Add another Class Library project to the current solution and name the project ClassLibrary2
(see Figure 15-22).
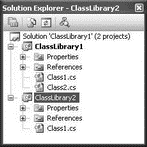
Figure 15-22
Populate the default Class1.cs
as follows:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace Learn2develop.net {
public class Class3 {
public void DoSomething() {
}
}
}
namespace CoolLabs.net {
public class Class5 {
public void DoSomething() {
}
}
}
This file contains two namespaces — Learn2develop.net
and CoolLabs.net
— each containing a class and a method.
Compile the ClassLibrary2
project so that an assembly is generated in the binDebug folder of the project — ClassLibrary2.dll
.
Now, add another Class Library project to the current solution, and this time use the Visual Basic language. Name the project ClassLibrary3
(see Figure 15-23).
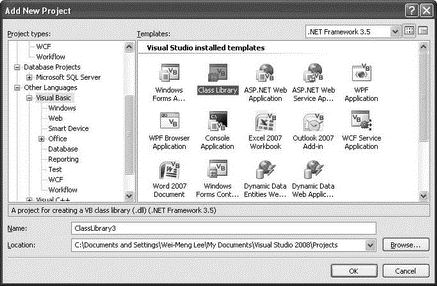
Figure 15-23
In the Properties page of the ClassLibrary3
project, set its root namespace to Learn2develop.net
(see Figure 15-24).
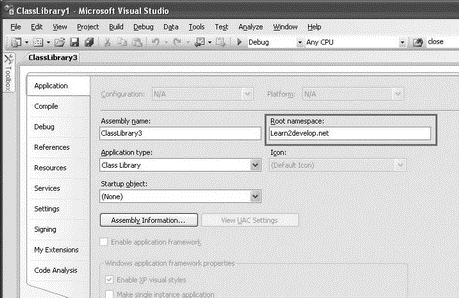
Figure 15-24
In the default Class1.vb
, define Class4
and add a method to it:
Public Class Class4
Public Sub DoSomething()
End Sub
End Class
Compile the ClassLibrary3
project so that an assembly is generated in the binDebug folder of the project — ClassLibrary3.dll
.
Now add a new Windows application project (name it WindowsApp
) to the current solution so that you can use the three assemblies (ClassLibrary1.dll
, ClassLibrary2.dll
, and ClassLibrary3.dll
) that you have created.
To use the three assemblies, you need to add a reference to all of them. Because the assemblies are created in the same solution as the current Windows project, you can find them in the Projects tab of the Add Reference dialog (see Figure 15-25).
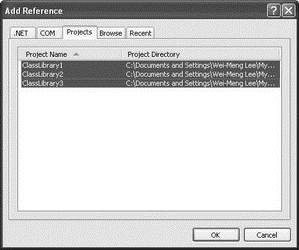
Figure 15-25
In the code-behind of the default Form1
, type the Learn2develop.net
namespace, and IntelliSense will show that four classes are available (see Figure 15-26).
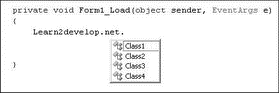
Figure 15-26
Even though the classes are located in different assemblies, IntelliSense still finds them because all these classes are grouped within the same namespace. You can now use the classes as follows:
Learn2develop.net.Class1 c1 = new Learn2develop.net.Class1();
c1.DoSomething();
Learn2develop.net.Class2 c2 = new Learn2develop.net.Class2();
c2.DoSomething();
Learn2develop.net.Class3 c3 = new Learn2develop.net.Class3();
c3.DoSomething();
Learn2develop.net.Class4 c4 = new Learn2develop.net.Class4();
c4.DoSomething();
For Class5
, you need to use the CoolLabs.net
namespace. If you don't, IntelliSense will check against all the referenced assemblies and suggest an appropriate namespace (see Figure 15-27).

Figure 15-27
You can use Class5
as follows:
CoolLabs.net.Class5 c5 = new CoolLabs.net.Class5();
c5.DoSomething();
Namespace Alias
There are times when you want to specify the fully qualified name of a class so that your code is easier to understand. For example, you usually import the namespace of a class and use the class like this:
using CoolLabs.net;
//...
Class5 c5 = Class5();
c5.DoSomething();
However, you might want to use the fully qualified name for Class5
to make it clear that Class5
belongs to the CoolLabs.net
namespace. To do so, you can rewrite your code like this:
CoolLabs.net.Class5 c5 = new CoolLabs.net.Class5();
c5.DoSomething();
But the CoolLabs.net namespace is quite lengthy and may make your code look long and unwieldy. To simplify the coding, you can give an alias to the namespace, like this:
using cl = CoolLabs.net;
//...
cl.Class5 c5 = cl.Class5();
c5.DoSomething();
Then, instead of using the full namespace, you can simply refer to the CoolLabs.net
namespace as cl
.
To summarize, this example shows that:
? Classes belonging to a specific namespace can be located in different assemblies.
? An assembly can contain one or more namespaces.
? Assemblies created using different languages are transparent to each other.
- Assemblies
- Разработка приложений баз данных InterBase на Borland Delphi
- Open Source Insight and Discussion
- Introduction to Microprocessors and Microcontrollers
- Chapter 6. Traversing of tables and chains
- Chapter 8. Saving and restoring large rule-sets
- Chapter 11. Iptables targets and jumps
- Chapter 5 Installing and Configuring VirtualCenter 2.0
- Chapter 16. Commercial products based on Linux, iptables and netfilter
- Appendix A. Detailed explanations of special commands
- Appendix B. Common problems and questions
- Appendix E. Other resources and links