Книга: C# 2008 Programmer
Extension Methods (C# 3.0)
Extension Methods (C# 3.0)
Whenever you add additional methods to a class in previous versions of C#, you need to subclass it and then add the required method. For example, consider the following predefined (meaning you cannot modify it) classes:
public abstract class Shape {
//---properties---
public double length { get; set; }
public double width { get; set; }
//---make this method as virtual---
public virtual double Perimeter() {
return 2 * (this.length + this.width);
}
//---abstract method---
public abstract double Area();
}
public class Rectangle : Shape {
public override sealed double Area() {
return this.length * this.width;
}
}
The only way to add a new method Diagonal()
to the Rectangle
class is to create a new class that derives from it, like this:
public class NewRectangle : Rectangle {
public double Diagonal() {
return Math.Sqrt(Math.Pow(this.length, 2) + Math.Pow(this.width, 2));
}
}
In C# 3.0, you just use the new extension method feature to add a new method to an existing type. To add the Diagonal()
method to the existing Rectangle
class, define a new static class and define the extension method (a static method) within it, like this:
public static class MethodsExtensions {
public static double Diagonal(this Rectangle rect) {
return Math.Sqrt(Math.Pow(rect.length, 2) + Math.Pow(rect.width, 2));
}
}
In this example, Diagonal()
is the extension method that is added to the Rectangle
class. You can use the Diagonal()
method just like a method from the Rectangle
class:
Rectangle r = new Rectangle();
r.length = 4;
r.width = 5;
//---prints out: 6.40312423743285---
Console.WriteLine(r.Diagonal());
The first parameter of an extension method is prefixed by the this
keyword, followed by the type it is extending (Rectangle in this example, indicating to the compiler that this extension method must be added to the Rectangle
class). The rest of the parameter list (if any) is then the signature of the extension method. For example, to pass additional parameters into the Diagonal()
extension method, you can declare it as:
public static double Diagonal(this Rectangle rect, int x, int y) {
//---additional implementation here---
return Math.Sqrt(Math.Pow(rect.length, 2) + Math.Pow(rect.width, 2));
}
To call this modified extension method, simply pass in two arguments, like this:
Console.WriteLine(r.Diagonal(3,4));
Figure 6-4 shows IntelliSense providing a hint on the parameter list.
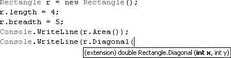
Figure 6-4
Although an extension method is a useful new feature in the C# language, use it sparingly. If an extension method has the same signature as another method in the class it is trying to extend, the method in the class will take precedence and the extension method will be ignored.