Книга: C# 2008 Programmer
Understanding Inheritance in C#
Understanding Inheritance in C#
The following Employee class contains information about employees in a company:
public class Employee {
public string Name { get; set; }
public DateTime DateofBirth { get; set; }
public ushort Age() {
return (ushort)(DateTime.Now.Year - this.DateofBirth.Year);
}
}
Manager is a class containing information about managers:
public class Manager {
public string Name { get; set; }
public DateTime DateofBirth { get; set; }
public ushort Age() {
return (ushort)(DateTime.Now.Year - this.DateofBirth.Year);
}
public Employee[] subordinates { get; set; }
}
The key difference between the Manager
class and the Employee
class is that Manager
has an additional property, subordinates
, that contains an array of employees under the supervision of a manager. In fact, a manager is actually an employee, except that he has some additional roles. In this example, the Manager
class could inherit from the Employee
class and then add the additional subordinates property that it requires, like this:
public class Manager: Employee {
public Employee[] subordinates { get; set; }
}
By inheriting from the Employee
class, the Manager class has all the members defined in the Employee
class made available to it. The relationships between the Employee
and Manager
classes can be represented using a class diagram as shown in Figure 6-1.
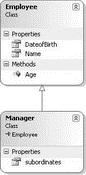
Figure 6-1
Employee
is known as the base class and Manager
is a derived class. In object-oriented programming, inheritance is classified into two types: implementation and interface. This chapter explores both.
- Chapter 6 Inheritance
- Implementation Inheritance
- Understanding the Command Line
- Understanding Set User ID and Set Group ID Permissions
- Understanding init Scripts and the Final Stage of Initialization
- Understanding Point-to-Point Protocol over Ethernet
- Understanding SQL Basics
- Understanding the Changes Made by DHCP
- Class Inheritance
- Multiple Inheritance
- Understanding Computer Attacks
- Understanding SELinux