Êíèãà: Advanced PIC Microcontroller Projects in C
PROJECT 7.4 — Temperature Logger
PROJECT 7.4 — Temperature Logger
This project shows the design of a temperature data logger system. The ambient temperature is read every ten seconds and stored in a file on an SD card. The program is menu-based, and the user is given the option of:
• Sending the saved file contents to a PC
• Saving the temperature readings to a new file on an SD card
• Appending the temperature readings to an existing file on an SD card
The hardware of this project is similar to the one for Project 7.1 (i.e., as shown in Figure 7.8), but here, in addition, the serial input port pin (RC7) is connected to the RS232 connector so data can be received from the PC keyboard. In addition, a LM35DZ-type analog temperature sensor is connected to the microcontroller’s analog input AN0 (pin 2). The new circuit diagram is shown in Figure 7.14.
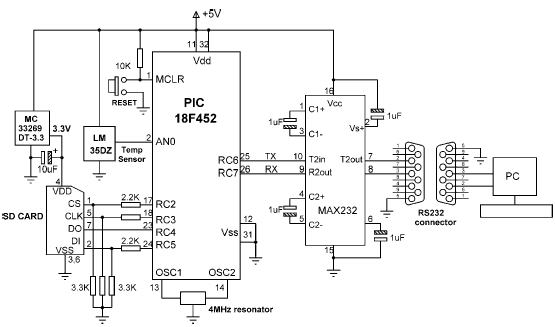
Figure 7.14: Circuit diagram of the project
The LM35 DZ is a three-pin analog temperature sensor that can measure with 1°C accuracy temperatures between 0°C and +100°C. One pin of the device is connected to the supply (+5V), another pin to the ground, and the third to the analog output. The output voltage of the sensor is directly proportional to the temperature (i.e., Vo=10mV/°C). If, for example, the temperature is 10°C, the output voltage will be 100mV, and if the temperature is 35°C, the output voltage of the sensor will be 350mV.
When the program is started, the following menu is displayed on the PC screen:
TEMPERATURE DATA LOGGER
1. Send temperature data to the PC
2. Save temperature data in a new file
3. Append temperature data to an existing file
Choice?
The user then chooses one of the three options. When an option is completed, the program does not return to the menu. To display the menu again the system has to be restarted.
The program listing of the project is shown in Figure 7.15 (program SD4.C). In this project, a file called TEMPERTRTXT is created on the SD card to store the temperature readings (the library function call will insert the “.” to make the filename “TEMPERTR.TXT”), if it does not already exist.
/**************************************************************
TEMPERATURE LOGGER PROJECT
============================
In this project a SD card is connected to PORTC as follows:
CS RC2
CLK RC3
DO RC4
DI RC5
In addition, a MAX232 type RS232 voltage level converter chip
is connected to serial ports RC6 and RC7. Also, a LM35DZ type
analog temperature sensor is connected to analog input AN0 of
the microcontroller.
The program is menu based. The user is given options of either
to send the saved temperature data to the PC, or to read and
save new data on the SD card, or to read temperature data and
append to the existing file. Temperature is read at every 10
seconds.
The temperature is stored in a file called TEMPERTR.TXT
Author: Dogan Ibrahim
Date: August 2007
File: SD4.C
**************************************************************/
char filename[] = "TEMPERTRTXT";
unsigned short character;
unsigned long file_size,i,rec_size;
unsigned char ch1,ch2,flag,ret_status,choice;
unsigned char temperature[10],txt[12];
//
// This function sends carriage-return and line-feed to USART
//
void Newline() {
Usart_Write(0x0D); // Send carriage-return
Usart_Write(0x0A); // Send line-feed
}
//
// This function sends a space character to USART
//
void Space() {
Usart_Write(0x20);
}
//
// This function sends a text to serial port
//
void Text_To_Usart(unsigned char *m) {
unsigned char i;
i = 0;
while(m[i] != 0) { // Send TEXT to serial port
Usart_Write(m[i]);
i++;
}
}
//
// This function reads the temperature from analog input AN0
//
void Get_Temperature() {
unsigned long Vin, Vdec,Vfrac;
unsigned char op[12];
unsigned char i,j;
Vin = Adc_Read(0); // Read from channel 0 (AN0)
Vin = 488*Vin; // Scale up the result
Vin = Vin /10; // Convert to temperature in C
Vdec = Vin / 100; // Decimal part
Vfrac = Vin % 100; // Fractional part
LongToStr(Vdec,op); // Convert Vdec to string in op
//
// Remove leading blanks
//
j=0;
for(i=0;i<=11;i++) {
if(op[i] != ' ') // If a blank
{
temperature[j]=op[i];
j++;
}
}
temperature[j] = '.'; // Add “.”
ch1 = Vfrac / 10; // fractional part
ch2 = Vfrac % 10;
j++;
temperature[j] = 48+ch1; // Add fractional part
j++;
temperature[j] = 48+ch2;
j++;
temperature[j] = 0x0D; // Add carriage-return
j++;
temperature[j] = 0x0A; // Add line-feed
j++;
temperature[j]='';
}
//
// Start of MAIN program
//
void main() {
rec_size = 0;
//
// Configure A/D converter
//
TRISA = 0xFF;
ADCON1 = 0x80;
// Use AN0, Vref = +5V
//
// Configure the serial port
//
Usart_Init(2400);
//
// Initialize the SPI bus
//
Spi_Init_Advanced(MASTER_OSC_DIV16, DATA_SAMPLE_MIDDLE,
CLK_IDLE_LOW, LOW_2_HIGH);
//
// Initialize the SD card bus
//
while(Mmc_Init(&PORTC,2));
//
// Initialize the FAT file system
//
while(Mmc_Fat_Init(&PORTC,2));
//
// Display the MENU and get user choice
//
Newline();
Text_To_Usart("TEMPERATURE DATA LOGGER");
Newline();
Newline();
Text_To_Usart("1. Send temperature data to the PC");
Newline();
Text_To_Usart("2. Save temperature data in a new file");
Newline();
Text_To_Usart("3. Append temperature data to an existing file");
Newline();
Newline();
Text_To_Usart("Choice ? ");
//
// Read a character from the PC keyboard
//
flag = 0;
do {
if (Usart_Data_Ready()) // If data received
{
choice = Usart_Read(); // Read the received data
Usart_Write(choice); // Echo received data
flag = 1;
}
} while (!flag);
Newline();
Newline();
//
// Now process user choice
//
switch(choice) {
case '1':
ret_status = Mmc_Fat_Assign(&filename,1);
if (!ret_status) {
Text_To_Usart("File does not exist..No saved data...");
Newline();
Text_To_Usart("Restart the program and save data to the file...");
Newline();
for(;;);
} else {
//
// Read the data and send to UART
//
Text_To_Usart("Sending saved data to the PC...");
Newline();
Mmc_Fat_Reset(&file_size);
for(i=0; i<file_size; i++) {
Mmc_Fat_Read(&character);
Usart_Write(character);
}
Newline();
text_To_Usart("End of data...");
Newline();
for(;;);
}
case '2':
//
// Start the A/D converter, get temperature readings every
// 10 seconds, and then save in a NEW file
//
Text_To_Usart("Saving data in a NEW file...");
Newline();
Mmc_Fat_Assign(&filename, 0x80); // Assign the file
Mmc_Fat_Rewrite(); // Clear
Mmc_Fat_Write("TEMPERATURE DATA - SAVED EVERY 10 SECONDSrn", 43);
//
// Read the temperature from A/D converter, format and save
//
for(;;) {
Mmc_Fat_Append();
Get_Temperature();
Mmc_Fat_Write(temperature,9);
rec_size++;
LongToStr(rec_size,txt);
Newline();
Text_To_Usart("Saving record:");
Text_To_Usart(txt);
Delay_ms(10000);
}
break;
case '3':
//
// Start the A/D converter, get temperature readings every
// 10 seconds, and then APPEND to the existing file
//
Text_To_Usart("Appending data to the existing file...");
Newline();
ret_status = Mmc_Fat_Assign(&filename,1); // Assign the file
if (!ret_status) {
Text_To_Usart("File does not exist - can not append...");
Newline();
Text_To_Usart("Restart the program and choose option 2...");
Newline();
for(;;);
} else {
//
// Read the temperature from A/D converter, format and save
//
for(;;) {
Mmc_Fat_Append();
Get_Temperature();
Mmc_Fat_Write(temperature,9);
rec_size++;
LongToStr(rec_size,txt);
Newline();
Text_To_Usart("Appending new record:");
Text_To_Usart(txt);
Delay_ms(10000);
}
}
default:
Text_To_Usart("Wrong choice...Restart the program and try again...");
Newline();
for(;;);
}
}

Figure 7.15: Program listing of the project
The following functions are created at the beginning of the program, before the main program:
Newline sends a carriage return and a line feed to the UART so the cursor moves to the next line.
Text_To_Usart receives a text string as its argument and sends it to the UART to display on the PC screen.
Get_Temperature starts the A/D conversion and receives the converted data into a variable called Vin. The voltage corresponding to this value is then calculated in millivolts and divided by 10 to find the actual measured temperature in °C. The decimal part of the found temperature is then converted into string form using function LongToStr. The leading spaces are removed from this string, and the resulting string is stored in character array temperature. Then the fractional parts of the measured temperature, a carriage return, and a line feed are added to this character array, which is later written to the SD card.
The following operations are performed inside the main program:
• Initialize the UART to 2400 baud
• Initialize the SPI bus
• Initialize the FAT file system
• Display menu on the PC screen
• Get a choice from the user (1, 2, or 3)
• If the choice = 1, assign the temperature file, read the temperature records, and display them on the PC screen
• If the choice = 2, create a new temperature file, get new temperature readings every ten seconds, and store them in the file
• If the choice = 3, assign to the temperature file, get new temperature readings every ten seconds, and append them to the existing temperature file
• If the choice is not 1, 2, or 3, display an error message on the screen The menu options are described here in more detail:
Option 1: The program attempts to assign the existing temperature file. If the file does not exist, the error messages “File does not exist…No saved data…” and “Restart the program and save data to the file…” are displayed on the screen, and the user is expected to restart the program. If, on the other hand, the temperature file already exists, then the message: “Sending saved data to the PC…” is displayed on the PC screen. Function Mmc_Fat_Reset is called to set the file pointer to the beginning of the file and also return the size of the file in bytes. Then a for loop is formed, temperature records are read from the card one byte at a time using function Mmc_Fat_Read, and these records are sent to the PC screen using function Usart_Write. At the end of the data the message “End of data…” is sent to the PC screen.
Option 2: In this option, the message “Saving data in a NEW file…” is sent to the PC screen, and a new file is created using function Mmc_Fat_Assign with the create flag set to 0x80. The message “TEMPERATURE DATA – SAVED EVERY 10 SECONDS” is written on the first line of the file using function Mmc_Fat_Write. Then, a for loop is formed, the SD card is set to file append mode by calling function Mmc_Fat_Append, and a new temperature reading is obtained by calling function Get_Temperature. The temperature is then written to the SD card. Also, the current record number appears on the PC screen to indicate that the program is actually working. This process is repeated after a ten-second delay.
Option 3: This option is very similar to Option 2, except that a new file is not created but rather the existing temperature file is opened in read mode. If the file does not exist, then an error message is displayed on the PC screen.
Default: If the user entry is a number other than 1, 2, or 3, then this option runs and displays the error message “Wrong choice…Restart the program and try again…” on the PC screen.
The project can be tested by connecting the output of the microcontroller to the serial port of a PC (e.g., COM1) and then running the HyperTerminal terminal emulation software. Set the communications parameters to 2400 baud, 8 data bits, 1 stop bit, and no parity bit. Figure 7.16 shows a snapshot of the PC screen when Option 2 is selected to save the temperature records in a new file. Notice that the current record numbers are displayed on the screen as they are written to the SD card.
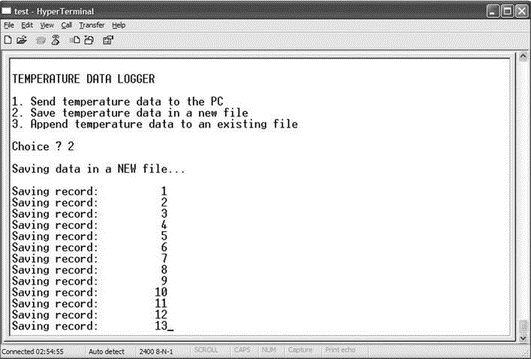
Figure 7.16: Saving temperature records on an SD card with Option 2
Figure 7.17 shows a screen snapshot where Option 1 is selected to read the temperature records from the SD card and display them on the PC screen.
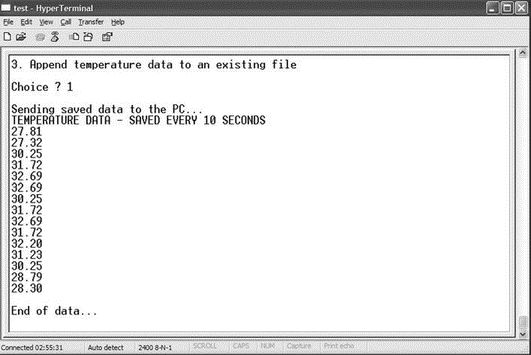
Figure 7.17: Displaying the records on the PC screen with Option 1
Finally, Figure 7.18 shows a screen snapshot when Option 3 is selected to append the temperature readings to the existing file.
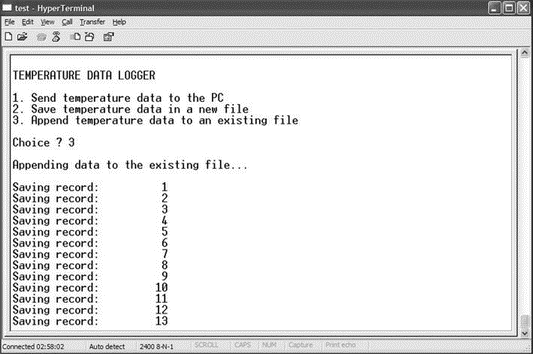
Figure 7.18: Saving temperature records on an SD card with Option 3
- CHAPTER 7 Advanced PIC18 Projects — SD Card Projects
- Project PDL
- Ãëàâà 5. Ðàçðàáîòêà è àíàëèç áèçíåñ-ïëàíîâ â ñèñòåìå Project Expert
- Turtle Firewall Project
- Testing the Project
- Using the C Programming Project Management Tools Provided with Fedora Linux
- Managing Software Projects with Subversion
- The Fedora Project
- Fedora Project Mailing Lists
- CHAPTER 6 Simple PIC18 Projects
- CHAPTER 8 Advanced PIC18 Projects — USB Bus Projects
- CHAPTER 9 Advanced PIC18 Projects — CAN Bus Projects