Книга: Beginning Android
Following the Script
Following the Script
Unlike other mobile-device operating systems, Android has no restrictions on what you can run on it, so long as you can do it in Java using the Dalvik VM. This includes incorporating your own scripting language into your application, something that is expressly prohibited on some other devices.
One possible Java scripting language is BeanShell.[26] BeanShell gives you Java-compatible syntax with implicit typing and no compilation required.
So, to add BeanShell scripting, you need to put the BeanShell interpreter’s JAR file in your libs/
directory. The 2.0b4 JAR available for download from the BeanShell site, unfortunately, does not work out of the box with the Android 0.9 and newer SDKs, perhaps due to the compiler that was used to build it. Instead, you should probably check out the source code from Subversion[27] and execute ant jarcore
to build it, then copy the resulting JAR (in BeanShell’s dist/
directory) to your own project’s libs/
. Or just use the BeanShell JAR that accompanies the source code for this book, in the Java/AndShell
project available in the Source Code area at http://apress.com.
From there, using BeanShell on Android is no different from using BeanShell in any other Java environment:
1. Create an instance of the BeanShell Interpreter
class.
2. Set any globals for the script’s use via Interpreter#set()
.
3. Call Interpreter#eval()
to run the script and, optionally, get the result of the last statement.
For example, here is the XML layout for the world’s smallest BeanShell IDE:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
>
<Button
android:id="@+id/eval"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="Go!"
/>
<EditText
android:id="@+id/script"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:singleLine="false"
android:gravity="top"
/>
</LinearLayout>
Couple that with the following activity implementation:
package com.commonsware.android.andshell;
import android.app.Activity;
import android.app.AlertDialog;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.Toast;
import bsh.Interpreter;
public class MainActivity extends Activity {
private Interpreter i = new Interpreter();
@Override
public void onCreate(Bundle icicle) {
super.onCreate(icicle);
setContentView(R.layout.main);
Button btn = (Button)findViewById(R.id.eval);
final EditText script = (EditText)findViewById(R.id.script);
btn.setOnClickListener(new View.OnClickListener() {
public void onClick(View view) {
String src = script.getText().toString();
try {
i.set("context", MainActivity.this);
i.eval(src);
} catch (bsh.EvalError e) {
AlertDialog.Builder builder =
new AlertDialog.Builder(MainActivity.this);
builder
.setTitle("Exception!").setMessage(e.toString())
.setPositiveButton("OK", null).show();
}
}
});
}
}
Compile and run it (including incorporating the BeanShell JAR as mentioned earlier), and install it on the emulator. Fire it up, and you get a trivial IDE with a large text area for your script and a big Go! button (see Figure 21-1) to execute it.
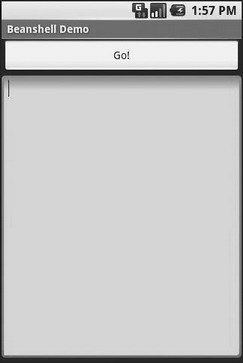
Figure 21-1. The AndShell BeanShell IDE
import android.widget.Toast;
Toast.makeText(context, "Hello, world!", 5000).show();
Note the use of context
to refer to the activity when making the Toast
. That is the global set by the activity to reference back to itself. You could call this global variable anything you want, so long as the set()
call and the script code use the same name.
When you click the Go! button, you get the result shown in Figure 21-2.
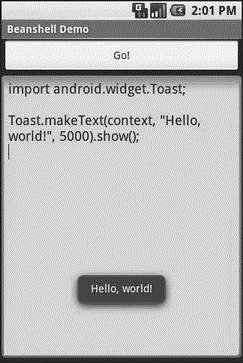
Figure 21-2. The AndShell BeanShell IDE, executing some code
And now, some caveats.
First, not all scripting languages will work. For example, those that implement their own form of just-in-time (JIT) compilation, generating Java bytecodes on the fly, would probably have to be augmented to generate Dalvik VM bytecodes instead of those for stock Java implementations. Simpler languages that execute from parsed scripts, calling Java reflection APIs to call back into compiled classes, will likely work better. Even there, though, not every feature of the language may work if the feature relies upon some facility in a traditional Java API that does not exist in Dalvik — for example, there could be stuff hidden inside BeanShell or the add-on JARs that does not work on today’s Android.
Second, scripting languages without JIT will inevitably be slower than compiled Dalvik applications. Slower may mean users experience sluggishness. Slower definitely means more battery life is consumed for the same amount of work. So, building a whole Android application in BeanShell, simply because you feel it is easier to program in may cause your users to be unhappy.
Third, scripting languages that expose the whole Java API, like BeanShell, can pretty much do anything the underlying Android security model allows. So, if your application has the READ_CONTACTS
permission, expect any BeanShell scripts your application runs to have the same permission.
Last, but certainly not least, is that language interpreter JARs tend to be… portly. The BeanShell JAR used in this example is 200KB. That is not ridiculous, considering what it does, but it will make applications that use BeanShell that much bigger to download, take up that much more space on the device, etc.
- The while Statement
- The Microcontroller Software
- The if and goto commands
- The login chat
- The checkgroups Message
- Saving and restoring the DHCP configuration
- 4.4.4 The Dispatcher
- About the author
- Chapter 7. The state machine
- Chapter 12. Debugging your scripts
- Chapter 14. Example scripts
- Appendix E. Other resources and links