Книга: C# 2008 Programmer
Handling Exceptions Using the try-catch Statement
Handling Exceptions Using the try-catch Statement
In C#, you can use the try-catch
statement to enclose a block of code statements that may potentially cause exceptions to be raised. You enclose these statements within the catch block and that block to catch the different types of exceptions that may occur.
Using the previous example, you can enclose the statements that ask the user to input num1
and num2
and then performs the division within a try
block. You then use the catch
block to catch possible exceptions, like this:
static void Main(string[] args) {
int num1, num2, result;
try {
Console.Write("Please enter the first number:");
num1 = int.Parse(Console.ReadLine());
Console.Write("Please enter the second number:");
num2 = int.Parse(Console.ReadLine());
result = num1 / num2;
Console.WriteLine("The result of {0}/{1} is {2}", num1, num2, result);
} catch (Exception ex) {
Console.WriteLine(ex.Message);
}
Console.ReadLine();
}
The Exception
class is the base class for all exceptions; that is, it catches all the various types of exceptions. The class contains the details of the exception that occurred, and includes a number of properties that help identify the code location, the type, the help file, and the reason for the exception. The following table describes these properties.
Property | Description |
---|---|
Data |
Gets a collection of key/value pairs that provide additional user-defined information about the exception. |
HelpLink |
Gets or sets a link to the help file associated with this exception. |
HResult |
Gets or sets HRESULT , a coded numerical value that is assigned to a specific exception. |
InnerException |
Gets the Exception instance that caused the current exception. |
Message |
Gets a message that describes the current exception. |
Source |
Gets or sets the name of the application or the object that causes the error. |
StackTrace |
Gets a string representation of the frames on the call stack at the time the current exception was thrown. |
TargetSite |
Gets the method that throws the current exception. |
In the preceding program, if you type in a numeric value for num1
and then an alphabetical character for num2
, the exception is caught and displayed like this:
Please enter the first number:6
Please enter the second number:a
Input string was not in a correct format.
If, though, you enter 0 for the second number, you get a different description for the error:
Please enter the first number:7
Please enter the second number:0
Attempted to divide by zero.
Notice that two different types of exceptions are caught using the same Exception
class. The description of the exception is contained within the Message
property of the Exception
class.
You can use the ToString()
method of the Exception
class to retrieve more details about the exception, such as the description of the exception as well as the stack trace.
However, there are cases where you would like to print your own custom error messages for the different types of exceptions. Using the preceding code, you would not be able to do that — you would need a much finer way to catch the different types of possible exceptions.
To know the different types of exceptions that your program can cause (such as entering "a" for num1
or division by zero), you can set a breakpoint at a line within the catch
block and try entering different values. When an exception is raised during runtime, IntelliSense tells you the error and the type of the exception raised. Figure 12-2 shows that the FormatException
exception is raised when you enter a for num1
.
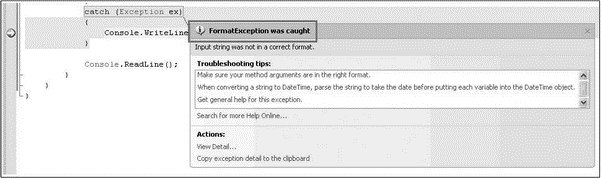
Figure 12-2
If you are not sure what type of exception your program is going to raise during runtime, it is always safe to use the base Exception
class. If not — if the exception that is raised does not match the exception you are trying to catch — a runtime error will occur. Here's an example:
static void Main(string[] args) {
int num1, num2, result;
try {
Console.Write("Please enter the first number:");
num1 = int.Parse(Console.ReadLine());
Console.Write("Please enter the second number:");
num2 = int.Parse(Console.ReadLine());
result = num1 / num2;
Console.WriteLine("The result of {0}/{1} is {2}", num1, num2, result);
} catch (DivideByZeroException ex) {
Console.WriteLine(ex.Message);
}
Console.ReadLine();
}
If a division-by-zero exception occurs (entering 0 for num2
), the exception is caught. However, if you enter an alphabetic character for num1
or num2
, a FormatException
exception is raised. And because you are only catching the DivideByZeroException
exception, this exception goes unhandled and a runtime error results.
- Handling Multiple Exceptions
- 4.4.4 The Dispatcher
- About the author
- Chapter 7. The state machine
- Appendix E. Other resources and links
- Caveats using NAT
- Example NAT machine in theory
- Using Double Quotes to Resolve Variables in Strings with Embedded Spaces
- The final stage of our NAT machine
- Compiling the user-land applications
- The conntrack entries
- Untracked connections and the raw table