Книга: C# 2008 Programmer
Exception Chaining
Exception Chaining
The InnerException
property is of type Exception
, and it can be used to store a list of previous exceptions. This is known as exception chaining.
To see how exception chaining works, consider the following program:
class Program {
static void Main(string[] args) {
Program myApp = new Program();
try {
myApp.Method1();
} catch (Exception ex) {
Console.WriteLine(ex.Message);
if (ex.InnerException != null)
Console.WriteLine(ex.InnerException.ToString());
}
Console.ReadLine();
}
private void Method1() {
try {
Method2();
} catch (Exception ex) {
throw new Exception(
"Exception caused by calling Method2() in Method1().", ex);
}
}
private void Method2() {
try {
Method3();
} catch (Exception ex) {
throw new Exception(
"Exception caused by calling Method3() in Method2().", ex);
}
}
private void Method3() {
try {
int num1 = 5, num2 = 0;
int result = num1 / num2;
} catch (DivideByZeroException ex) {
throw new Exception("Division by zero error in Method3().", ex);
}
}
}
In this program, the Main()
function calls Method1()
, which in turns calls Method2()
. Method2()
then calls Method3()
. In Method3(),
a division-by-zero exception occurs and you rethrow a new Exception
exception by passing in the current exception (DividebyZeroException
). This exception is caught by Method2()
, which rethrows a new Exception
exception by passing in the current exception. Method1()
in turn catches the exception and rethrows a new Exception
exception. Finally, the Main()
function catches the exception and prints out the result as shown in Figure 12-4.
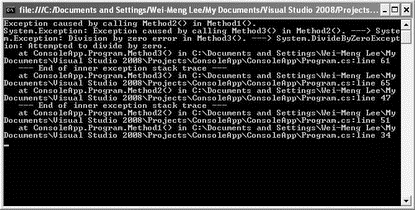
Figure 12-4
If you set a breakpoint in the catch block within the Main()
function, you will see that the InnerException
property contains details of each exception and that all the exceptions are chained via the InnerException
property (see Figure 12-5).
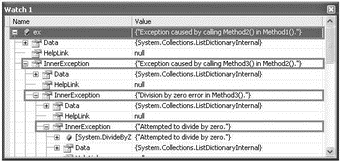
Figure 12-5
- Chapter 12 Exception Handling
- Delegates Chaining (Multicast Delegates)
- Handling Exceptions
- Exception Handling
- Выражения в EXCEPTION
- 3.2.5.2 Lock chaining
- 9.2.4. Traps, Exceptions, and Interrupts
- Chapter 10: Exceptions and Interrupts
- 10.2 What are Exceptions and Interrupts?
- 10.3 Applications of Exceptions and Interrupts
- 10.4 A Closer Look at Exceptions and Interrupts
- 10.4.2 Classification of General Exceptions