Книга: C# 2008 Programmer
Escape Characters
Escape Characters
Certain characters have special meaning in strings. For example, strings are always enclosed in double quotation marks, and if you want to use the actual double-quote character in the string, you need to tell the C# compiler by "escaping" the character's special meaning. For instance, say you need to represent the following in a string:
"I don't necessarily agree with everything I say." Marshall McLuhan
Because the sentence contains the double-quote characters, simply using a pair of double- quotes to contain it will cause an error:
//---error--- string quotation;
quotation = ""I don't necessarily agree with everything I say." Marshall McLuhan";
To represent the double-quote character in a string, you use the backslash () character to turn off its special meanings, like this:
string quotation =
""I don't necessarily agree with everything I say." Marshall McLuhan";
Console.WriteLine(quotation);
The output is shown in Figure 8-1.

Figure 8-1
A backslash, then, is another special character. To represent the C:Windows path, for example, you need to turn off the special meaning of by using another , like this:
string path = "C:Windows";
What if you really need two backslash characters in your string, as in the following?
"servernamepath"
In that case, you use the backslash character twice, once for each of the backslash characters you want to turn off, like this:
string UNC = "\servernamepath";
In addition to using the character to turn off the special meaning of characters like the double-quote (") and backslash (), there are other escape characters that you can use in strings.
One common escape character is the n. Here's an example:
string lines = "Line 1nLine 2nLine 3nLine 4nLine 5";
Console.WriteLine(lines);
The n escape character creates a newline, as Figure 8-2 shows.
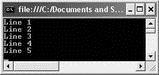
Figure 8-2
You can also use t
to insert tabs into your string, as the following example shows (see also Figure 8-3):
string columns1 = "Column 1tColumn 2tColumn 3tColumn 4";
string columns2 = "1t5t25t125";
Console.WriteLine(columns1);
Console.WriteLine(columns2);

Figure 8-3
You learn more about formatting options in the section "String Formatting" later in this chapter.
Besides the n and t
escape characters, C# also supports the r
escape character. r
is the carriage return character. Consider the following example:
string str1 = " One";
string str2 = "Two";
Console.Write(str1);
Console.Write(str2);
The output is shown in Figure 8-4.
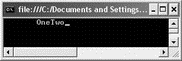
Figure 8-4
However, if you prefix a r
escape character to the beginning of str2
, the effect will be different:
string str1 = " One";
string str2 = "rTwo";
Console.Write(str1);
Console.Write(str2);
The output is shown in Figure 8-5.
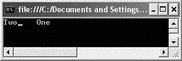
Figure 8-5
The r
escape character simply brings the cursor to the beginning of the line, and hence in the above statements the word "Two
" is printed at the beginning of the line. The r
escape character is often used together with n to form a new line (see Figure 8-6):
string str1 = "Line 1nr";
string str2 = "Line 2nr";
Console.Write(str1);
Console.Write(str2);
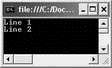
Figure 8-6
By default, when you use the n to insert a new line, the cursor is automatically returned to the beginning of the line. However, some legacy applications still require you to insert newline and carriage return characters in strings.
The following table summarizes the different escape sequences you have seen in this section:
Sequence | Purpose |
---|---|
n | New line |
r |
Carriage return |
rn |
Carriage return; New line |
" |
Quotation marks |
|
Backslash character |
t |
Tab |
In C#, strings can also be @-quoted. Earlier, you saw that to include special characters (such as double-quote, backslash, and so on) in a string you need to use the backslash character to turn off its special meaning:
string path="C:Windows";
You can actually use the @
character, and prefix the string with it, like this:
string path=@"C:Windows";
Using the @
character makes your string easier to read. Basically, the compiler treats strings that are prefixed with the @ character verbatim — that is, it just accepts all the characters in the string (inside the quotes). To better appreciate this, consider the following example where a string containing an XML snippet is split across multiple lines (with each line ending with a carriage return):
string XML = @"
<Books>
<title>C# 3.0 Programmers' Reference</title>
</Book>";
Console.WriteLine(XML);
Figure 8-7 shows the output. The WriteLine()
method prints out the line verbatim.
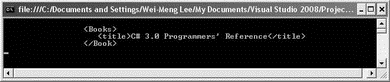
Figure 8-7
To illustrate the use of the @ character on a double-quoted string, the following:
string quotation =
""I don't necessarily agree with everything I say." Marshall McLuhan";
Console.WriteLine(quotation);
can be rewritten as:
string quotation =
@"""I don't necessarily agree with everything I say."" Marshall McLuhan";
Console.WriteLine(quotation);
Escape Code for Unicode
C# supports the use of escape code to represent Unicode characters. The four-digit escape code format is: udddd
. For example, the following statement prints out the ? symbol:
string symbol = "u00A3";
Console.WriteLine(symbol);
For more information on Unicode, check out http://unicode.org/Public/UNIDATA/NamesList.txt.