Книга: Advanced PIC Microcontroller Projects in C
COLLECTOR Program
COLLECTOR Program
Figure 9.18 shows the program listing of the COLLECTOR program, called COLLECTOR.C. The initial part of this program is the same as the DISPLAY program. The receive filter is set to 500 so that messages with identifier 500 are accepted by the program.
/***********************************************************************
CAN BUS EXAMPLE - NODE: COLLECTOR
=================================
This is the COLLECTOR node of the CAN bus example. In this project a
PIC18F258 type microcontroller is used. An MCP2551 type CAN bus transceiver
is used to connect the microcontroller to the CAN bus. The microcontroller is
operated from an 8MHz crystal with an external reset button.
Pin CANRX and CANTX of the microcontroller are connected to pins RXD
and TXD of the transceiver chip respectively. Pins CANH and CANL of the
transceiver chip are connected to the CAN bus.
An LM35DZ type analog temperature sensor is connected to port AN0 of the
microcontroller. The microcontroller reads the temperature when a request is
received and then sends the temperature value as a byte to Node:DISPLAY on
the CAN bus.
CAN speed parameters are:
Microcontroller clock: 8MHz
CAN Bus bit rate: 100Kb/s
Sync_Seg: 1
Prop_Seg: 6
Phase_Seg1: 6
Phase_Seg2: 7
SJW: 1
BRP: 1
Sample point: 65%
Author: Dogan Ibrahim
Date: October 2007
File: COLLECTOR.C
**********************************************************************/
void main() {
unsigned char temperature, data[8];
unsigned short init_flag, send_flag, dt, len, read_flag;
char SJW, BRP, Phase_Seg1, Phase_Seg2, Prop_Seg, txt[4];
unsigned int temp;
unsigned long mV;
long id, mask;
TRISA = 0xFF; // PORTA are inputs
TRISB = 0x08; // RB2 is output, RB3 is input
//
// Configure A/D converter
//
ADCON1 = 0x80;
//
// CAN BUS Timing Parameters
//
SJW = 1;
BRP = 1;
Phase_Seg1 = 6;
Phase_Seg2 = 7;
BRP = 1;
Prop_Seg = 6;
init_flag = CAN_CONFIG_SAMPLE_THRICE &
CAN_CONFIG_PHSEG2_PRG_ON &
CAN_CONFIG_STD_MSG &
CAN_CONFIG_DBL_BUFFER_ON &
CAN_CONFIG_VALID_XTD_MSG &
CAN_CONFIG_LINE_FILTER_OFF;
send_flag = CAN_TX_PRIORITY_0 &
CAN_TX_XTD_FRAME &
CAN_TX_NO_RTR_FRAME;
read_flag = 0;
//
// Initialise CAN module
//
CANInitialize(SJW, BRP, Phase_Seg1, Phase_Seg2, Prop_Seg, init_flag);
//
// Set CAN CONFIG mode
//
CANSetOperationMode(CAN_MODE_CONFIG, 0xFF);
mask = -1;
//
// Set all MASK1 bits to 1's
//
CANSetMask(CAN_MASK_B1, mask, CAN_CONFIG_XTD_MSG);
//
// Set all MASK2 bits to 1's
//
CANSetMask(CAN_MASK_B2, mask, CAN_CONFIG_XTD_MSG);
//
// Set id of filter B1_F1 to 3
//
CANSetFilter(CAN_FILTER_B2_F3,500,CAN_CONFIG_XTD_MSG);
//
// Set CAN module to NORMAL mode
//
CANSetOperationMode(CAN_MODE_NORMAL, 0xFF);
//
// Program loop. Read the temperature from analog temperature
// sensor
//
for(;;) // Endless loop
{
//
// Wait until a request is received
//
dt = 0;
while(!dt) dt = CANRead(&id, data, &len, &read_flag);
if (id == 500 && data[0] == 'T') {
//
// Now read the temperature
//
temp = Adc_Read(0); // Read temp
mV = (unsigned long)temp * 5000 / 1024; // in mV
temperature = mV/10; // in degrees C
//
// send the temperature to Node:Display
//
data[0] = temperature;
id = 3; // Identifier
CANWrite(id, data, 1, send_flag); // send temperature
}
}
}

Figure 9.18: COLLECTOR program listing
Inside the program loop, the program waits until it receives a request to send the temperature. Here the request is identified by the reception of character “T”. Once a valid request is received, the temperature is read and converted into °C (stored in variable temperature) and then sent to the CAN bus as a byte with an identifier value equal to 3. This process repeats forever.
Figure 9.19 summarizes the operation of both nodes.
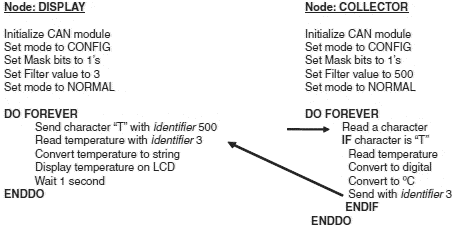
Figure 9.19: Operation of both nodes
- The COLLECTOR Processor
- DISPLAY Program
- 2. How to Apply These Terms to Your New Programs
- The Programmers
- Для чего нужны папки Windows, Documents and Settings, Program Files и Temp?
- Можно ли указать использование по умолчанию вместо C:Program Files другого каталога для установки программ?
- Можно ли удалять из папки Program Files папки деинсталлированных программ?
- 6.1 Program Description Language (PDL)
- The Simplest Windows Program
- The Generic Windows Program
- PART V Programming Linux
- The GNU Image Manipulation Program