Книга: Beginning Android
Parsing Responses
Parsing Responses
The response you get will be formatted using some system — HTML, XML, JSON, whatever. It is up to you, of course, to pick out what information you need and do something useful with it. In the case of the WeatherDemo
, we need to extract the forecast time, temperature, and icon (indicating sky conditions and precipitation) and generate an HTML page from it.
Android includes:
• Three XML parsers: the traditional W3C DOM (org.w3c.dom
), a SAX parser (org.xml.sax
), and the XML pull parser discussed in Chapter 19
• A JSON parser (org.json
)
You are also welcome to use third-party Java code, where possible, to handle other formats, such as a dedicated RSS/Atom parser for a feed reader. The use of third-party Java code is discussed in Chapter 21.
For WeatherDemo
, we use the W3C DOM parser in our buildForecasts()
method:
void buildForecasts(String raw) throws Exception {
DocumentBuilder builder = DocumentBuilderFactory
.newInstance().newDocumentBuilder();
Document doc = builder.parse(new InputSource(new StringReader(raw)));
NodeList times = doc.getElementsByTagName("start-valid-time");
for (int i=0; i<times.getLength(); i++) {
Element time = (Element)times.item(i);
Forecast forecast = new Forecast();
forecasts.add(forecast);
forecast.setTime(time.getFirstChild().getNodeValue());
}
NodeList temps = doc.getElementsByTagName("value");
for (int i=0; i<temps.getLength(); i++) {
Element temp = (Element)temps.item(i);
Forecast forecast = forecasts.get(i);
forecast.setTemp(new Integer(temp.getFirstChild().getNodeValue()));
}
NodeList icons = doc.getElementsByTagName("icon-link");
for (int i=0; i<icons.getLength(); i++) {
Element icon = (Element)icons.item(i);
Forecast forecast = forecasts.get(i);
forecast.setIcon(icon.getFirstChild().getNodeValue());
}
}
The National Weather Service XML format is… curiously structured, relying heavily on sequential position in lists versus the more object-oriented style you find in formats like RSS or Atom. That being said, we can take a few liberties and simplify the parsing somewhat, taking advantage of the fact that the elements we want (start-valid-time
for the forecast time, value for the temperature, and icon-link
for the icon URL) are all unique within the document.
The HTML comes in as an InputStream
and is fed into the DOM parser. From there, we scan for the start-valid-time
elements and populate a set of Forecast
models using those start times. Then, we find the temperature value elements and icon-link
URLs and fill those into the Forecast
objects.
In turn, the generatePage()
method creates a rudimentary HTML table with the forecasts:
String generatePage() {
StringBuffer bufResult = new StringBuffer("<html><body><table>");
bufResult.append("<tr><th width="50%">Time</th>" +
"<th>Temperature</th><th>Forecast</th></tr>");
for (Forecast forecast : forecasts) {
bufResult.append("<tr><td align="center">");
bufResult.append(forecast.getTime());
bufResult.append("</td><td align="center">");
bufResult.append(forecast.getTemp());
bufResult.append("</td><td><img src="");
bufResult.append(forecast.getIcon());
bufResult.append(""></td></tr>");
}
bufResult.append("</table></body></html>");
return(bufResult.toString());
}
The result can be seen in Figure 22-1.
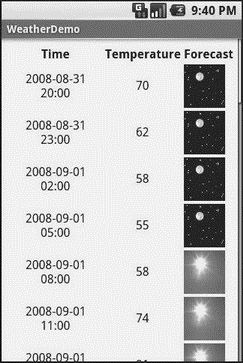
Figure 22-1. The WeatherDemo sample application