Книга: Beginning Android
XML: The Resource Way
XML: The Resource Way
In Chapter 18, we showed how you can package XML files as raw resources and get access to them for parsing and usage. There is another way of packaging static XML with your application: the XML resource.
Simply put the XML file in res/xml/
, and you can access it by getXml()
on a Resources object, supplying it a resource ID of R.xml.
plus the base name of your XML file. So, in an activity, with an XML file of words.xml
, you could call getResources().getXml(R.xml.words)
.
This returns an instance of the currently-undocumented XmlPullParser
, found in the org.xmlpull.v1
Java namespace. Documentation for this library can be found at the parser’s site[17] as of this writing.
An XML pull parser is event-driven: you keep calling next()
on the parser to get the next event, which could be START_TAG
, END_TAG
, END_DOCUMENT
, etc. On a START_TAG
event, you can access the tag’s name and attributes; a single TEXT
event represents the concatenation of all text nodes that are direct children of this element. By looping, testing, and invoking per-element logic, you parse the file.
To see this in action, let’s rewrite the Java code for the Files/Static
sample project to use an XML resource. This new project, Resources/XML
, requires that you place the words.xml
file from Static
not in res/raw/
, but in res/xml/
. The layout stays the same, so all that needs replacing is the Java source:
package com.commonsware.android.resources;
import android.app.Activity;
import android.os.Bundle;
import android.app.ListActivity;
import android.view.View;
import android.widget.AdapterView;
import android.widget.ArrayAdapter;
import android.widget.ListView;
import android.widget.TextView;
import android.widget.Toast;
import java.io.InputStream;
import java.util.ArrayList;
import org.xmlpull.v1.XmlPullParser;
import org.xmlpull.v1.XmlPullParserException;
public class XMLResourceDemo extends ListActivity {
TextView selection;
ArrayList<String> items = new ArrayList<String>();
@Override
public void onCreate(Bundle icicle) {
super.onCreate(icicle);
setContentView(R.layout.main);
selection = (TextView)findViewById(R.id.selection);
try {
XmlPullParser xpp = getResources().getXml(R.xml.words);
while (xpp.getEventType()!=XmlPullParser.END_DOCUMENT) {
if (xpp.getEventType()==XmlPullParser.START_TAG) {
if (xpp.getName().equals("word")) {
items.add(xpp.getAttributeValue(0));
}
}
xpp.next();
}
} catch (Throwable t) {
Toast
.makeText(this, "Request failed: " + t.toString(), 4000).show();
}
setListAdapter(new ArrayAdapter<String>(this,
android.R.layout.simple_list_item_1, items));
}
public void onListItemClick(ListView parent, View v, int position,
long id) {
selection.setText(items.get(position).toString());
}
}
Now, inside our try...catch
block, we get our XmlPullParser
and loop until the end of the document. If the current event is START_TAG
and the name of the element is word xpp.getName().equals("word"))
, then we get the one-and-only attribute and pop that into our list of items for the selection widget. Since we’re in complete control over the XML file, it is safe enough to assume there is exactly one attribute. But, if you were not as comfortable that the XML is properly defined, you might consider checking the attribute count (getAttributeCount())
and the name of the attribute (getAttributeName()
) before blindly assuming the 0-index attribute is what you think it is.
As you can see in Figure 19-4, the result looks the same as before, albeit with a different name in the title bar.
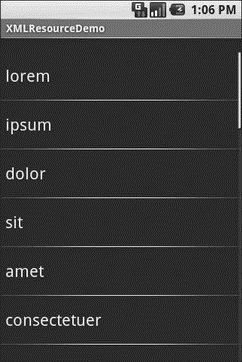
Figure 19-4. The XMLResourceDemo sample application
- Тестирование Web-сервиса XML с помощью WebDev.WebServer.exe
- Преобразование XML в реляционную базу данных
- 4.4.4 The Dispatcher
- About the author
- Chapter 7. The state machine
- Appendix E. Other resources and links
- Example NAT machine in theory
- The final stage of our NAT machine
- Compiling the user-land applications
- The conntrack entries
- Untracked connections and the raw table
- Basics of the iptables command