Книга: Beginning Android
A Browser, Writ Small
A Browser, Writ Small
For simple stuff, WebView is not significantly different than any other widget in Android — pop it into a layout, tell it what URL to navigate to via Java code, and you’re done.
For example, WebKit/Browser1
is a simple layout with a WebView. You can find WebKit/Browser1
along with all the code samples for this chapter in the Source Code area at http://apress.com.
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
>
<WebView android:id="@+id/webkit"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
/>
</LinearLayout>
As with any other widget, you need to tell it how it should fill up the space in the layout (in this case, it fills all remaining space).
The Java code is equally simple:
package com.commonsware.android.webkit;
import android.app.Activity;
import android.os.Bundle;
import android.webkit.WebView;
public class BrowserDemo1 extends Activity {
WebView browser;
@Override
public void onCreate(Bundle icicle) {
super.onCreate(icicle);
setContentView(R.layout.main);
browser = (WebView)findViewById(R.id.webkit);
browser.loadUrl("http://commonsware.com");
}
}
The only unusual bit with this edition of onCreate()
is that we invoke loadUrl()
on the WebView
widget, to tell it to load a Web page (in this case, the home page of some random firm).
However, we also have to make one change to AndroidManifest.xml
, requesting permission to access the Internet:
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.commonsware.android.webkit">
<uses-permission android:name="android.permission.INTERNET" />
<application>
<activity android:name=".BrowserDemo1" android:label="BrowserDemo1">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
If we fail to add this permission, the browser will refuse to load pages.
The resulting activity looks like a Web browser, just with hidden scrollbars (see Figure 13-1).
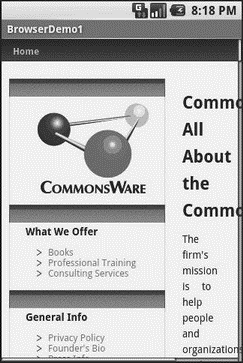
Figure 13-1. The Browser1 sample application
As with the regular Android browser, you can pan around the page by dragging it, while the directional pad moves you around all the focusable elements on the page.
What is missing is all the extra accouterments that make up a Web browser, such as a navigational toolbar.
Now, you may be tempted to replace the URL in that source code with something else, such as Google’s home page or another page that relies upon Javascript. By default Javascript is turned off in WebView
widgets. If you want to enable Javascript, call getSettings().setJavaScriptEnabled(true);
on the WebView
instance.
- CHAPTER 13 Embedding the WebKit Browser
- Forced writes - палка о двух концах
- Forced Writes
- Why this document was written
- How it was written
- Working with OpenOffice.org Writer
- Productivity Applications Written for Microsoft Windows
- Листинг 14.2. Использование параметра XMLWriteMode при сохранении объекта ADO.NET DataSet
- CHAPTER 27 Writing PHP Scripts
- CHAPTER 33 Writing and Executing a Shell Script
- 13.3. cbrowser
- Глава 3 Работа с ПО интерактивной доски Interwrite Board