Книга: C# 2008 Programmer
Attributes
Разделы на этой странице:
Attributes
Attributes are descriptive tags that can be used to provide additional information about types (classes), members, and properties. Attributes can be used by .NET to decide how to handle objects while an application is running.
There are two types of attributes:
? Attributes that are defined in the CLR.
? Custom attributes that you can define in your code.
CLR Attributes
Consider the following Contact
class definition:
class Contact {
public string FirstName;
public string LastName;
public void PrintName() {
Console.WriteLine("{0} {1}", this.FirstName, this.LastName);
}
[Obsolete("This method is obsolete. Please use PrintName()")]
public void PrintName(string FirstName, string LastName) {
Console.WriteLine("{0} {1}", FirstName, LastName);
}
}
Here, the PrintName()
method is overloaded — once with no parameter and again with two input parameters. Notice that the second PrintName()
method is prefixed with the Obsolete
attribute:
[Obsolete("This method is obsolete. Please use PrintName()")]
That basically marks the method as one that is not recommended for use. The class will still compile, but when you try to use this method, a warning will appear (see Figure 4-7).
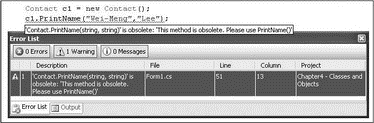
Figure 4-7
The Obsolete
attribute is overloaded — if you pass in true
for the second parameter, the message set in the first parameter will be displayed as an error (by default the message is displayed as a warning):
[Obsolete("This method is obsolete. Please use PrintName()", true)]
Figure 4-8 shows the error message displayed when you use the PrintName()
method marked with the Obsolete
attribute with the second parameter set to true
.
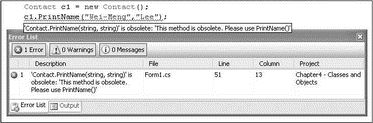
Figure 4-8
Attributes can also be applied to a class. In the following example, the Obsolete
attribute is applied to the Contact
class:
[Obsolete("This class is obsolete. Please use NewContact")]
class Contact {
//...
}
Custom Attributes
You can also define your own custom attributes. To do so, you just need to define a class that inherits directly from System.Attribute
. The following Programmer
class is one example of a custom attribute:
public class Programmer : System.Attribute {
private string _Name;
public double Version;
public string Dept { get; set; }
public Programmer(string Name) {
this._Name = Name;
}
}
In this attribute, there are:
? One private member (_Name
)
? One public member (Version
)
? One constructor, which takes in one string argument
Here's how to apply the Programmer
attribute to a class:
[Programmer("Wei-Meng Lee", Dept="IT", Version=1.5)]
class Contact {
//...
}
You can also apply the Programmer
attribute to methods (as the following code shows), properties, structure, and so on:
[Programmer("Wei-Meng Lee", Dept="IT", Version=1.5)]
class Contact {
[Programmer("Jason", Dept = "CS", Version = 1.6)]
public void PrintName() {
Console.WriteLine("{0} {1}", this.FirstName, this.LastName);
}
//...
}
Use the AttributeUsage
attribute to restrict the use of any attribute to certain types:
[System.AttributeUsage(System.AttributeTargets.Class |
System.AttributeTargets.Method |
System.AttributeTargets.Property)]
public class Programmer : System.Attribute {
private string _Name;
public double Version;
public string Dept { get; set; }
public Programmer(string Name) {
this._Name = Name;
}
}
In this example, the Programmer
attribute can only be used on class definitions, methods, and properties.