Книга: C# 2008 Programmer
Your First WCF Service
Your First WCF Service
Developing a WCF service using Visual Studio 2008 will be helpful in comparing it with the traditional ASMX Web Services.
Using Visual Studio 2008, create a new WCF Service Library application, and name it WcfServiceLibraryTest
(see Figure 20-7).
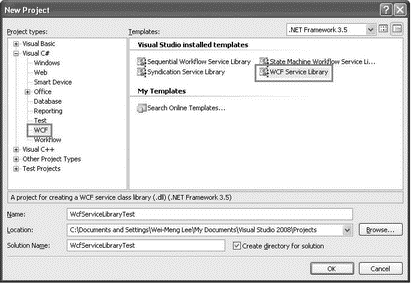
Figure 20-7
Notice the two files created in the project (see Figure 20-8):
? iService1.cs
contains the service contract as well as the data contract.
? Service1.cs
contains the implementation of the contract defined in the IService1.cs
file.
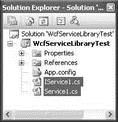
Figure 20-8
Here's the content of the IService1.cs
file:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Runtime.Serialization;
using System.ServiceModel;
using System.Text;
namespace WcfServiceLibraryTest {
// NOTE: If you change the interface name "IService1" here, you must also
// update the reference to "IService1" in App.config.
[ServiceContract]
public interface IService1 {
[OperationContract]
string GetData(int value);
[OperationContract]
CompositeType GetDataUsingDataContract(CompositeType composite);
// TODO: Add your service operations here
}
// Use a data contract as illustrated in the sample below to add composite
// types to service operations
[DataContract]
public class CompositeType {
bool boolValue = true;
string stringValue = "Hello ";
[DataMember]
public bool BoolValue {
get { return boolValue; }
set { boolValue = value; }
}
[DataMember]
public string StringValue {
get { return stringValue; }
set { stringValue = value; }
}
}
}
Here, there is an interface (IService1
) and a class (CompositeType
) defined. The IService1
interface is set with the [ServiceContract]
attribute to indicate that this is a service contract and contains signatures of operations exposed by the service. Within this interface are signatures of methods that you will implement in the Service1.cs
file. Each method is set with the [OperationContract]
attribute to indicate that it is an operation. If you have additional operations to add, you can add them here.
The CompositeType
class is prefixed with the [DataContract]
attribute. This class defines the various composite data types required by your service.
The Service1.cs
file contains the implementation for the operations defined in the IService1
interface in the IService1.cs
file:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Runtime.Serialization;
using System.ServiceModel;
using System.Text;
namespace WcfServiceLibraryTest {
// NOTE: If you change the class name "Service1" here, you must also update the
// reference to "Service1" in App.config.
public class Service1 : IService1 {
public string GetData(int value) {
return string.Format("You entered: {0}", value);
}
public CompositeType GetDataUsingDataContract(CompositeType composite) {
if (composite.BoolValue) {
composite.StringValue += "Suffix";
}
return composite;
}
}
}
For now, use the default implementation provided by Visual Studio 2008 and examine how the service works.
Press F5 to debug the service. A WCF Test Client window will be displayed (see Figure 20-9). This is a test client shipped with Visual Studio 2008 to help you test your WCF service.
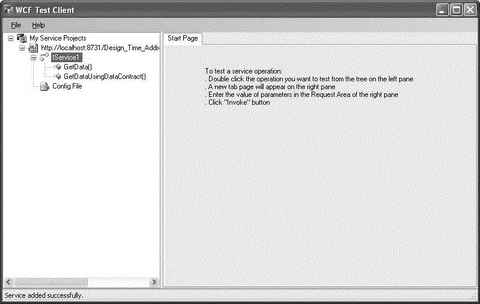
Figure 20-9
Expand the IService1
item, and select the GetData()
method. In the right of the window, enter 5 for the value and click the Invoke button (see Figure 20-10).
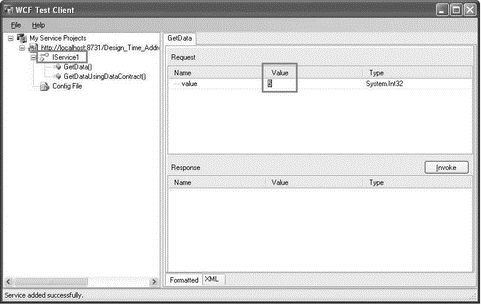
Figure 20-10
When you see a security warning dialog, click OK. The service returns its result in the Response pane (see Figure 20-11).
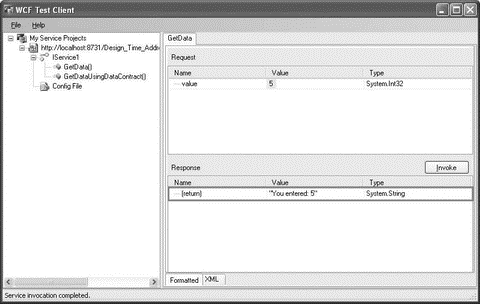
Figure 20-11
Also, try the GetDataUsingDataContract()
operation and enter some values as shown in Figure 20-12. Click Invoke, and observe the results returned.
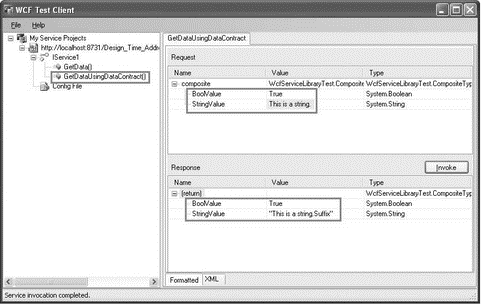
Figure 20-12
You can also see the SOAP messages exchanged between the test client and the service by clicking on the XML tab (see Figure 20-13).
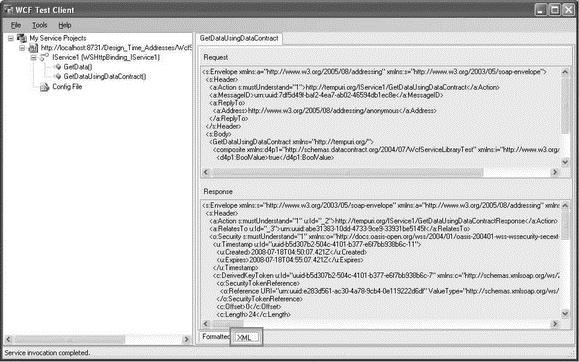
Figure 20-13
Notice that the SOAP messages contain a lot more information than a traditional ASMX Web Service SOAP packet. This is because WCF services, by default, use wsHttpBinding
, which ensures that information exchanged between the client and the service is encrypted automatically.
You'll see more about wsHttpBinding
later in this chapter.
Close the WCF Test Client window. Back in Visual Studio 2008, edit the IService1.cs
file, adding the getAge()
function signature to the IService1
interface:
[ServiceContract]
public interface IService1 {
[OperationContract]
string GetData(int value);
[OperationContract]
CompositeType GetDataUsingDataContract(CompositeType composite);
[OperationContract]
int getAge(Contact c);
}
By default, the [OperationContract]
attribute specifies a request/response messaging pattern for the operation.
After the class definition for CompositeType
, define the following data contract called Contact
:
[DataContract]
public class CompositeType {
//...
}
[DataContract]
public class Contact {
[DataMember]
public string Name { get; set; }
[DataMember]
public int YearofBirth { get; set; }
}
In Service1.cs
, define the getAge()
function as follows:
public class Service1 : IService1 {
//...
//...
public int getAge(Contact c) {
return (DateTime.Now.Year - c.YearofBirth);
}
}
Press F5 to test the application again. This time, select the getAge()
method, enter your name and year of birth, and then click Invoke (see Figure 20-14). Observe the result returned by the service.
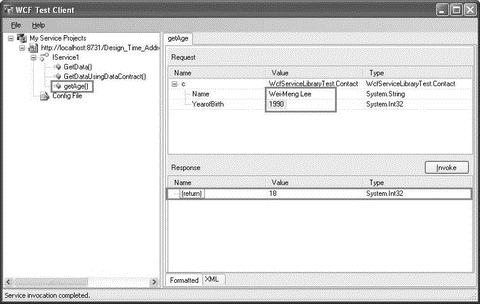
Figure 20-14
- Ограничение результатов выборки FIRST
- Chapter 12. Debugging your scripts
- Listing your active rule-set
- Updating and flushing your tables
- Internet Service Providers who use assigned IP addresses
- How to use this License for your documents
- 2. How to Apply These Terms to Your New Programs
- Получение помощи по Windows SharePoint Services 3.0
- Чтобы установить Service Pack 2, надо ли предварительно устанавливать Service Pack 1?
- Я установил Service Pack 2 для Windows XP, но с ним не хотят работать некоторые программы. Как его удалить из системы?
- Можно ли интегрировать в пакет установки Windows Service Pack и другие обновления, чтобы потом не приходилось их устанав...
- Система просит вставить диск Windows XP и Windows XP Service Pack 2 CD. Но ведь диск с дистрибутивом Windows у меня один...