Книга: C# 2008 Programmer
Starting a Thread
Starting a Thread
You can use threads to break up statements in your application into smaller chunks so that they can be executed in parallel. You could, for instance, use a separate thread to call the DoSomething()
function in the preceding example and let the remaining of the code continue to execute.
Every application contains one main thread of execution. A multithreaded application contains two or more threads of execution.
In C#, you can create a new thread of execution by using the Thread
class found in the System.Threading
namespace. The Thread
class creates and controls a thread. The constructor of the Thread
class takes in a ThreadStart
delegate, which wraps the function that you want to run as a separate thread. The following code shows to use the Thread
class to run the DoSomething()
function as a separate thread:
Import the System.Threading
namespace when using the Thread
class.
class Program {
static void Main(string[] args) {
Thread t = new Thread(new ThreadStart(DoSomething));
t.Start();
Console.WriteLine("Continuing with the execution...");
Console.ReadLine();
}
static void DoSomething() {
while (true) {
Console.WriteLine("Doing something...");
}
}
}
Note that the thread is not started until you explicitly call the Start()
method. When the Start()
method is called, the DoSomething()
function is called and control is immediately returned to the Main()
function. Figure 10-2 shows the output of the example application.
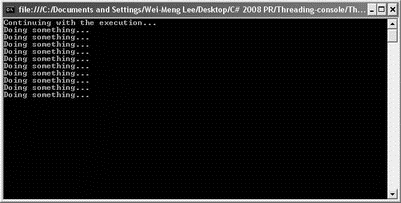
Figure 10-2
Figure 10-3 shows graphically the two different threads of execution.
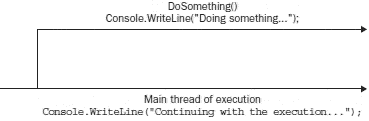
Figure 10-3
As shown in Figure 10-2, it just so happens that before the DoSomething()
method gets the chance to execute, the main thread has proceeded to execute its next statements. Hence, the output shows the main thread executing before the DoSomething()
method. In reality, both threads have an equal chance of executing, and one of the many possible outputs could be:
Doing something...
Doing something...
Continuing with the execution...
Doing something...
Doing something...
...
A thread executes until:
? It reaches the end of its life (method exits), or
? You prematurely kill (abort) it.
- 15.4.2. Debugging Multithreaded Applications
- The Need for Multithreading
- Класс System.Threading.Thread
- Starting SNAT and the POSTROUTING chain
- 6.6.5 SIGEV_THREAD
- 4 A few ways to use threads
- Starting the Install
- Starting X
- Starting X from the Console by Using startx
- Starting and Stopping Services Manually
- Starting and Stopping Apache
- Starting the Apache Server Manually