Книга: C# 2008 Programmer
Generic Classes
Generic Classes
Using generics, you do not need to fix the data type of the items used by your stack class. Instead, you use a generic type parameter (<T>
) that identifies the data type parameter on a class, structure, interface, delegate, or procedure. Here's a rewrite of the MyStack
class that shows the use of generics:
public class MyStack<T> {
private T[] _elements;
private int _pointer;
public MyStack(int size) {
_elements = new T[size];
_pointer = 0;
}
public void Push(T item) {
if (_pointer > _elements.Length - 1) {
throw new Exception("Stack is full.");
}
_elements[_pointer] = item;
_pointer++;
}
public T Pop() {
_pointer--;
if (_pointer < 0) {
throw new Exception("Stack is empty.");
}
return _elements[_pointer];
}
}
As highlighted, you use the type T
as a placeholder for the eventual data type that you want to use for the class. In other words, during the design stage of this class, you do not specify the actual data type that the MyStack
class will deal with. The MyStack
class is now known as a generic type.
When declaring the private member array _element
, you use the generic parameter T
instead of a specific type such as int
or string
:
private T[] _elements;
In short, you replace all specific data types with the generic parameter T
.
You can use any variable name you want to represent the generic parameter. T
is chosen as the generic parameter for illustration purposes.
If you want the MyStack
class to manipulate items of type int
, specify that during the instantiation stage (int
is called the type argument):
MyStack<int> stack = new MyStack<int>(3);
The stack object is now known as a constructed type, and you can use the MyStack
class normally:
stack.Push(1);
stack.Push(2);
stack.Push(3);
A constructed type is a generic type with at least one type argument.
In Figure 9-1 IntelliSense shows that the Push()
method now accepts arguments of type int
.
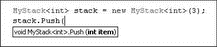
Figure 9-1
Trying to push a string value into the stack like this:
stack.Push("A"); //---Error---
generates a compile-time error. That's because the compiler checks the data type used by the MyStack
class during compile time. This is one of the key advantages of using generics in C#.
To use the MyStack
class for String
data types, you simply do this:
MyStack<string> stack = new MyStack<string>(3);
stack.Push("A");
stack.Push("B");
stack.Push("C");
Figure 9-2 summarizes the terms used in a generic type.
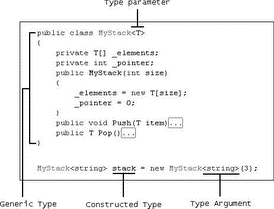
Figure 9-2
- Classes
- Understanding Generics
- Advantages of Generics
- Generic Interfaces
- Generic Structs
- Generic Methods
- Generic Delegates
- Generics and the .NET Framework Class Library
- 16. Object Reorientation: Generic Functions
- 17. Object Reorientation: Classes
- Generic Functions and Classes
- SCTP Generic header format