Книга: Advanced PIC Microcontroller Projects in C
PROJECT 10.3 — Voltmeter with RS232 Serial Output
In this RTOS project, which is more complex than the preceding ones, the voltage is read using an A/D converter and then sent over the serial port to a PC. The project consists of three tasks: Live, Get_voltage, and To_RS232.
• Task Live runs every 200ms and flashes the LED connected to port pin RD7. This LED indicates that the system is working.
• Task Generator runs every millisecond and increments a byte variable called count continuously. When the push-button switch is pressed, pin 0 of PORTD (RD0) goes to logic 0. When this happens, the current value of count is sent to task Display using RTOS function call rtos_msg_send(display, count), where Display is the name of the task where the message is sent and count is the byte sent.
• Task Display runs every 10ms. This task checks whether there is a message in the queue. If so, the message is extracted using RTOS function call rtos_msg_read(), and the read byte is sent to the LEDs connected to PORTB. Thus, the LEDs display the binary value of count as the switch is pressed. The message queue should be checked by using function rtos_msg_poll(), as trying to read the queue without any bytes in the queue may freeze the program.
In this RTOS project, which is more complex than the preceding ones, the voltage is read using an A/D converter and then sent over the serial port to a PC. The project consists of three tasks: Live, Get_voltage, and To_RS232.
Figure 10.12 shows the block diagram of the project. The circuit diagram is given in Figure 10.13. A PIC18F8520-type microcontroller with a 10MHz crystal is used in this project (though any PIC18F-series microcontroller can be used). The voltage to be measured is connected to analog port AN0 of the microcontroller. The RS232 TX output of the microcontroller (RC6) is connected to a MAX232-type RS232-level converter chip and then to the serial input of a PC (e.g., COM1) using a 9-pin D-type connector. Port pin RD7 is connected to an LED to indicate whether the project is working.
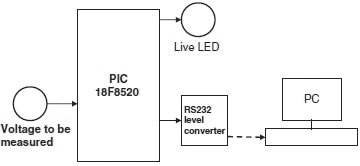
Figure 10.12: Block diagram of the project
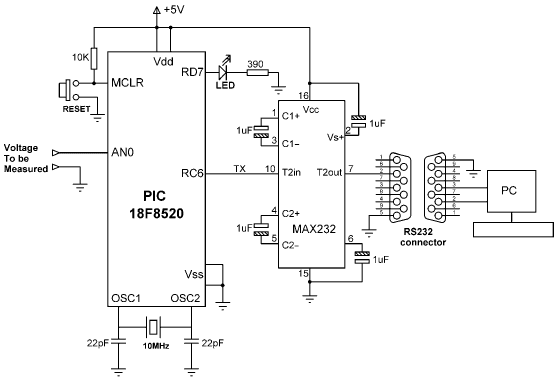
Figure 10.13: Circuit diagram of the project
The program listing (RTOS3.C) of the project is given in Figure 10.14. At the beginning of the program, the A/D is defined as 10 bits, the clock is defined as 10MHz, and the RS232 speed is defined as 2400 baud. The RTOS timer and the minor_cycle are then defined using the #use rtos preprocessor command.
//////////////////////////////////////////////////////////////////////////////
//
// SIMPLE RTOS EXAMPLE - VOLTMETER WITH RS232 OUTPUT
// ---------------------------------------------------------------------------
//
// This is a simple RTOS example. Analog voltage to be measured (between 0V
// and +5V) is connected to analog input AN0 of a PIC18F8520 type
// microcontroller. The microcontroller is operated from a 10MHz crystal. In
// addition, an LED is connected to port in RD7 of the microcontroller.
//
// RS232 serial output of the mirocontroller (RC6) is connected to a MAX232
// type RS232 voltage level converter chip. The output of this chip can be
// connected to the serial input of a PC (e.g., COM1) so that the measured
// voltage can be seen on the PC screen.
//
// The program consists of 3 tasks called "live", "Get_voltage", and
// "To_RS232".
//
// Task "Live" runs every 200ms and it flashes the LED connected to port pin
// RD7 of the microcontroller to indicate that the program is running and is
// ready to measure voltages.
//
// task "Get_voltage" reads analog voltage from port AN0 and then converts
// the voltage into millivolts and stores in a variable called Volts.
//
// Task "To_RS232" gets the measured voltage, converts it into a character
// array and then sends to the PC over the RS232 serial line. The serial line
// is configured to operate at 2400 Baud (higher Baud rates can also be used
// if desired).
//
// Programmer: Dogan Ibrahim
// Date: September, 2007
// File: RTOS3.C
//
//////////////////////////////////////////////////////////////////////////////
#include <18F8520.h>
#device adc=10
#use delay (clock=10000000)
#use rs232(baud=2400,xmit=PIN_C6,rcv=PIN_C7)
unsigned int16 adc_value;
unsigned int32 Volts;
//
// Define which timer to use and minor_cycle for RTOS
//
#use rtos(timer=0, minor_cycle=100ms)
//
// Declare TASK "Live" - called every 200ms
// This task flashes the LED on port RD7
//
#task(rate=200ms, max=1ms)
void Live() {
output_toggle(PIN_D7); // Toggle RD7 LED
}
//
// Declare TASK "Get_voltage" - called every 10ms
//
#task(rate=2s, max=100ms)
void Get_voltage() {
adc_value = read_adc(); // Read A/D value
Volts = (unsigned int32)adc_value*5000;
Volts = Volts / 1024; // Voltage in mV
}
//
// Declare TASK "To_RS232" - called every millisecond
//
#task(rate=2s, max=100ms)
void To_RS232() {
printf("Measured Voltage = %LumVnr",Volts); // send to RS232
}
//
// Start of MAIN program
//
void main() {
set_tris_d(0); // PORTD all outputs
output_d(0); // Clear PORTD
set_tris_a(0xFF); // PORTA all inputs
setup_adc_ports(ALL_ANALOG); // A/D ports
setup_adc(ADC_CLOCK_DIV_32); // A/D clock
set_adc_channel(0); // Select channel 0 (AN0)
delay_us(10);
rtos_run(); // Start RTOS
}

Figure 10.14: Program listing of the project
In the main part of the program PORTD is configured as output and all PORTD pins are cleared. Then PORTA is configured as input (RA0 is the analog input), the microcontroller’s analog inputs are configured, the A/D clock is set, and the A/D channel 0 is selected (AN0). The RTOS is then started by calling function rtos_run().
The program consists of three tasks:
• Task Live runs every 200ms and flashes an LED connected to port pin RD7 of the microcontroller to indicate that the project is working.
• Task Get_voltage reads the analog voltage from channel 0 (pin RA0 or AN0) of the microcontroller. The value is then converted into millivolts by multiplying by 5000 and dividing by 1024 (in a 10-bit A/D there are 1024 quantization levels, and when working with a reference voltage of +5V, each quantization level corresponds to 5000/1024mV). The voltage is stored in a global variable called Volts.
• Task To_RS232 reads the measured voltage from common variable Volts and sends it to the RS232 port using the C printf statement. The result is sent in the following format:
Measured voltage = nnnn mV
The HyperTerminal program is run on the PC to get an output from the program. A typical screen output is shown in Figure 10.15.
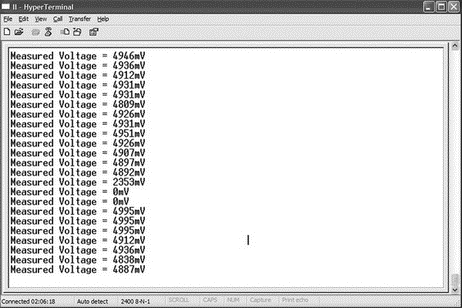
Figure 10.15: Typical output from the program
- 7.2.9. Цепочка OUTPUT
- Project PDL
- Using Double Quotes to Resolve Variables in Strings with Embedded Spaces
- Drawbacks with restore
- Binary Serialization
- OUTPUT chain
- Глава 5. Разработка и анализ бизнес-планов в системе Project Expert
- Turtle Firewall Project
- 7. AGGREGATION WITH INDEPENDENT WORKS
- Testing the Project
- Конструкция with-do
- 3. Hexadecimal – the way we communicate with micros