Книга: C# 2008 Programmer
#define and #undef
#define and #undef
The #define
preprocessor directive allows you to define a symbol so that you can use the #if
preprocessor directive to evaluate and then make conditional compilation. To see how the #define preprocessor directive works, assume that you have a console application named TestDefine
(saved in C:) created using Visual Studio 2008 (see Figure 3-12).
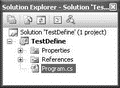
Figure 3-12
The Main() method is located in the Program.cs file. The program basically asks the user to enter a number and then sums up all the odd number from 1 to that number:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace TestDefine {
class Program {
static void Main(string[] args) {
Console.Write("Please enter a number: ");
int num = int.Parse(Console.ReadLine());
int sum = 0;
for (int i = 1; i <= num; i++) {
//---sum up all odd numbers---
if (i % 2 == 1) sum += i;
}
Console.WriteLine(
"Sum of all odd numbers from 1 to {0} is {1}",
num, sum);
Console.ReadLine();
}
}
}
Suppose that you want to add some debugging statements to the program so that you can print out the intermediate results. The additional lines of code are highlighted:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace TestDefine {
class Program {
static void Main(string[] args) {
Console.Write("Please enter a number: ");
int num = int.Parse(Console.ReadLine());
int sum = 0;
for (int i = 1; i <= num; i++) {
//---sum up all odd numbers---
if (i % 2 == 1)
{
sum += i;
Console.WriteLine("i={0}, sum={1}", i, sum);
}
}
Console.WriteLine(
"Sum of all odd numbers from 1 to {0} is {1}",
num, sum);
Console.ReadLine();
}
}
}
You do not want the debugging statements to be included in the production code so you first define a symbol (such as DEBUG
) using the #define
preprocessor directive and wrap the debugging statements with the #if
and #endif
preprocessor directives:
#define DEBUG
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace TestDefine {
class Program {
static void Main(string[] args) {
Console.Write("Please enter a number: ");
int num = int.Parse(Console.ReadLine());
int sum = 0;
for (int i = 1; i <= num; i++) {
//---sum up all odd numbers---
if (i % 2 == 1) {
sum += i;
#if DEBUG
Console.WriteLine("i={0}, sum={1}", i, sum);
#endif
}
}
Console.WriteLine(
"Sum of all odd numbers from 1 to {0} is {1}",
num, sum);
Console.ReadLine();
}
}
}
DEBUG
is a common symbol that developers use to indicate debugging statements, which is why most books use it in examples. However, you can define any symbol you want using the #define
preprocessor directive.
Before compilation, the preprocessor will evaluate the #if
preprocessor directive to see if the DEBUG
symbol has been defined. If it has, the statement(s) wrapped within the #if
and #endif
preprocessor directives will be included for compilation. If the DEBUG
symbol has not been defined, the statement — the statement(s) wrapped within the #if
and #endif
preprocessor — will be omitted from the compilation.
To test out the TestDefine
program, follow these steps:
1. Launch the Visual Studio 2008 command prompt (Start?Programs?Microsoft Visual Studio 2008?Visual Studio Tools?Visual Studio 2008 Command Prompt).
2. Change to the path containing the program (C:TestDefine).
3. Compile the application by issuing the command:
csc Program.cs
4. Run the program by issuing the command:
Program.exe
Figure 3-13 shows the output of the application. As you can see, the debugging statement prints out the intermediate results.
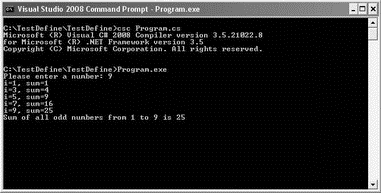
Figure 3-13
To undefine a symbol, you can use the #undef
preprocessor directive, like this:
#undef DEBUG
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
...
If you recompile the program now, the debugging statement will be omitted.
Another popular way of using the #define
preprocessor directive is to omit the definition of the symbol and inject it during compilation time. For example, if you remove the #define
preprocessor directive from the program, you can define it using the /define
compiler option:
1. In Visual Studio 2008 command prompt, compile the program using:
csc Program.cs /define:DEBUG
2. Run the program by issuing the command:
Program.exe
The output is identical to what you saw in Figure 3-13 — the debugging statement prints out the intermediate results.
If you now recompile the program by defining another symbol (other than DEBUG), you will realize that the debugging output does not appear (see Figure 3-14).
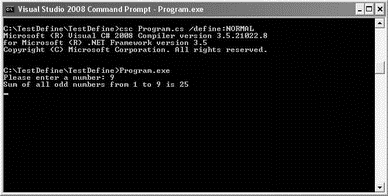
Figure 3-14
- Разработка приложений баз данных InterBase на Borland Delphi
- Open Source Insight and Discussion
- Introduction to Microprocessors and Microcontrollers
- Chapter 6. Traversing of tables and chains
- Chapter 8. Saving and restoring large rule-sets
- Chapter 11. Iptables targets and jumps
- Chapter 5 Installing and Configuring VirtualCenter 2.0
- Chapter 16. Commercial products based on Linux, iptables and netfilter
- Appendix A. Detailed explanations of special commands
- Appendix B. Common problems and questions
- Appendix E. Other resources and links
- IP filtering terms and expressions