Книга: C# 2008 Programmer
Enumerations
Enumerations
You can create your own set of named constants by using enumerations. In C#, you define an enumeration by using the enum
keyword. For example, say that you need a variable to store the day of a week (Monday, Tuesday, Wednesday, and so on):
static void Main(string[] args) {
int day = 1; //--- 1 to represent Monday---
//...
Console.ReadLine();
return;
}
In this case, rather than use a number to represent the day of a week, it would be better if the user could choose from a list of possible named values representing the days in a week. The following code example declares an enumeration called Days
that comprises seven names (Sun, Mon, Tue, and so forth). Each name has a value assigned (Sun is 0, Mon is 1, and so on):
namespace HelloWorld {
public enum Days {
Sun = 0,
Mon = 1,
Tue = 2,
Wed = 3,
Thur = 4,
Fri = 5,
Sat = 6
}
class Program {
static void Main(string[] args) {
Days day = Days.Mon;
Console.WriteLine(day); //---Mon---
Console.WriteLine((int) day); //---1---
Console.ReadLine();
return;
}
}
}
Instead of representing the day of a week using an int variable, you can create a variable of type Days
. Visual Studio 2008's IntelliSense automatically displays the list of allowed values in the Days
enumeration (see Figure 3-9).
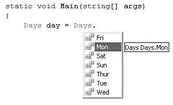
Figure 3-9
By default, the first value in an enumerated type is zero. However, you can specify a different initial value, such as:
public enum Ranking {
First = 100,
Second = 50,
Third = 25
}
To print out the value of an enumerated type, you can use the ToString()
method to print out its name, or typecast the enumerated type to int
to obtain its value:
Console.WriteLine(day); //---Mon---
Console.WriteLine(day.ToString()); //---Mon---
Console.WriteLine((int)day); //---1---
For assigning a value to an enumerated type, you can either use the name directly or typecast the value to the enumerated type:
Days day;
day = (Days)3; //---Wed---
day = Days.Wed; //---Wed---