Книга: C# 2008 Programmer
LINQ and Anonymous Types
LINQ and Anonymous Types
Although Chapter 4 explored anonymous types and how they allow you to define data types without having to formally define a class, you have not yet seen their real use. In fact, anonymous type is another new feature that Microsoft has designed with LINQ in mind.
Consider the following Contact
class definition:
public class Contact {
public int id { get; set; }
public string FirstName { get; set; }
public string LastName { get; set; }
}
Suppose that you have a list containing Contact
objects, like this:
List<Contact> Contacts = new List<Contact>() {
new Contact() {id = 1, FirstName = "John", LastName = "Chen"},
new Contact() {id = 2, FirstName = "Maryann", LastName = "Chen" },
new Contact() {id = 3, FirstName = "Richard", LastName = "Wells" }
};
You can use LINQ to query all contacts with Chen
as the last name:
IEnumerable<Contact> foundContacts =
from c in Contacts
where c.LastName == "Chen"
select c;
The foundContacts
object is of type IEnumerable<Contact>
. To print out all the contacts in the result, you can use the foreach
loop:
foreach (var c in foundContacts) {
Console.WriteLine("{0} - {1} {2}", c.id, c.FirstName, c.LastName);
}
The output looks like this:
1 - John Chen
2 - Maryann Chen
However, you can modify your query such that the result can be shaped into a custom class instead of type Contact
. To do so, modify the query as the following highlighted code shows:
var foundContacts =
from c in Contacts
where c.LastName == "Chen"
select new {
id = c.id,
Name = c.FirstName + " " + c.LastName
};
Here, you reshape the result using the anonymous type feature new in C# 3.0. Notice that you now have to use the var
keyword to let the compiler automatically infer the type of foundContacts
. Because the result is an anonymous type that you are defining, the following generates an error:
IEnumerable<Contact> foundContacts =
from c in Contacts
where c.LastName == "Chen"
select new {
id = c.id,
Name = c.FirstName + " " + c.LastName
};
To print the results, use the foreach
loop as usual:
foreach (var c in foundContacts) {
Console.WriteLine("{0} - {1}", c.id, c.Name);
}
Figure 14-4 shows that IntelliSense automatically knows that the result is an anonymous type with two fields — id
and Name
.
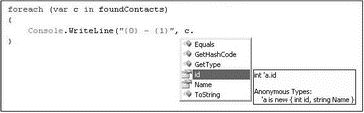
Figure 14-4
- Разработка приложений баз данных InterBase на Borland Delphi
- Open Source Insight and Discussion
- Introduction to Microprocessors and Microcontrollers
- Chapter 6. Traversing of tables and chains
- Chapter 8. Saving and restoring large rule-sets
- Chapter 11. Iptables targets and jumps
- Chapter 5 Installing and Configuring VirtualCenter 2.0
- Chapter 16. Commercial products based on Linux, iptables and netfilter
- Appendix A. Detailed explanations of special commands
- Appendix B. Common problems and questions
- Appendix C. ICMP types
- Appendix E. Other resources and links