Книга: C# 2008 Programmer
Working with Files Using the File and FileInfo Classes
Разделы на этой странице:
Working with Files Using the File and FileInfo Classes
The .NET Framework class library contains two similar classes for dealing with files — FileInfoand
File.
The File
class provides static methods for creating, deleting, and manipulating files, whereas the FileInfo
class exposes instance members for files manipulation.
Like the Directory
class, the File
class only exposes static methods and does not contain any properties.
Consider the following program, which creates, deletes, copies, renames, and sets attributes in files, using the File
class:
static void Main(string[] args) {
string filePath = @"C:temptextfile.txt";
string fileCopyPath = @"C:temptextfile_copy.txt";
string newFileName = @"C:temptextfile_newcopy.txt";
try {
//---if file already existed---
if (File.Exists(filePath)) {
//---delete the file---
File.Delete(filePath);
}
//---create the file again---
FileStream fs = File.Create(filePath);
fs.Close();
//---make a copy of the file---
File.Copy(filePath, fileCopyPath);
//--rename the file---
File.Move(fileCopyPath, newFileName);
//---display the creation time---
Console.WriteLine(File.GetCreationTime(newFileName));
//---make the file read-only and hidden---
File.SetAttributes(newFileName, FileAttributes.ReadOnly);
File.SetAttributes(newFileName, FileAttributes.Hidden);
} catch (IOException ex) {
Console.WriteLine(ex.Message);
} catch (Exception ex) {
Console.WriteLine(ex.Message);
}
Console.ReadLine();
}
This program first checks to see if a file exists by using the Exists()
method. If the file exists, the program deletes it using the Delete()
method. It then proceeds to create the file by using the Create()
method, which returns a FileStream
object (more on this in subsequent sections). To make a copy of the file, you use the Copy()
method. The Move()
method moves a file from one location to another. Essentially, you can use the Move()
method to rename a file. Finally, the program sets the ReadOnly
and Hidden
attribute to the newly copied file.
In addition to the File class, you have the FileInfo
class that provides instance members for dealing with files. Once you have created an instance of the FileInfo
class, you can use its members to obtain more information about a particular file. Figure 11-1 shows the different methods and properties exposed by an instance of the FileInfo
class, such as the Attributes
property, which retrieves the attributes of a file, the Delete()
method that allows you to delete a file, and so on.
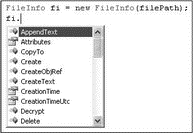
Figure 11-1
Reading and Writing to Files
The File
class contains four methods to write content to a file:
? WriteAllText()
— Creates a file, writes a string to it, and closes the file
? AppendAllText()
— Appends a string to an existing file
? WriteAllLines()
— Creates a file, writes an array of string to it, and closes the file
? WriteAllBytes()
— Creates a file, writes an array of byte to it, and closes the file
The following statements show how to use the various methods to write some content to a file:
string filePath = @"C:temptextfile.txt";
string strTextToWrite = "This is a string";
string[] strLinesToWrite = new string[] { "Line1", "Line2" };
byte[] bytesToWrite =
ASCIIEncoding.ASCII.GetBytes("This is a string");
File.WriteAllText(filePath, strTextToWrite);
File.AppendAllText(filePath, strTextToWrite);
File.WriteAllLines(filePath, strLinesToWrite);
File.WriteAllBytes(filePath, bytesToWrite);
The File
class also contains three methods to read contents from a file:
? ReadAllText()
— Opens a file, reads all text in it into a string, and closes the file
? ReadAllLines()
— Opens a file, reads all the text in it into a string array, and closes the file
? ReadAllBytes()
— Opens a file, reads all the content in it into a byte array, and closes the file
The following statements show how to use the various methods to read contents from a file:
string filePath = @"C:temptextfile.txt";
string strTextToRead = (File.ReadAllText(filePath));
string[] strLinestoRead = File.ReadAllLines(filePath);
byte[] bytesToRead = File.ReadAllBytes(filePath);
The beauty of these methods is that you need not worry about opening and closing the file after reading or writing to it; they close the file automatically after they are done.
StreamReader and StreamWriter Classes
When dealing with text files, you may also want to use the StreamReader
and StreamWriter
classes. StreamReader
is derived from the TextReader
class, an abstract class that represents a reader that can read a sequential series of characters.
You'll see more about streams in the "The Stream Class" section later in this chapter.
The following code snippet uses the StreamReader
class to read lines from a text file:
try {
using (StreamReader sr = new StreamReader(filePath)) {
string line;
while ((line = sr.ReadLine()) != null) {
Console.WriteLine(line);
}
}
} catch (Exception ex) {
Console.WriteLine(ex.ToString());
}
In addition to the ReadLine()
method, the StreamReader
class supports the following methods:
? Read()
— Reads the next character from the input stream
? ReadBlock()
— Reads a maximum of specified characters
? ReadToEnd()
— Reads from the current position to the end of the stream
The StreamWriter
class is derived from the abstract TextWriter
class and is used for writing characters to a stream. The following code snippet uses the StreamWriter
class to write lines to a text file:
try {
using (StreamWriter sw = new StreamWriter(filePath)) {
sw.Write("Hello, ");
sw.WriteLine("World!");
}
} catch (Exception ex) {
Console.WriteLine(ex.ToString());
}
BinaryReader and BinaryWriter Classes
If you are dealing with binary files, you can use the BinaryReader
and BinaryWriter
classes. The following example reads binary data from one file and writes it into another, essentially making a copy of the file:
string filePath = @"C:tempVS2008Pro.png";
string filePathCopy = @"C:tempVS2008Pro_copy.png";
//---open files for reading and writing---
FileStream fs1 = File.OpenRead(filePath);
FileStream fs2 = File.OpenWrite(filePathCopy);
BinaryReader br = new BinaryReader(fs1);
BinaryWriter bw = new BinaryWriter(fs2);
//---read and write individual bytes---
for (int i = 0; i <= br.BaseStream.Length - 1; i++)
bw.Write(br.ReadByte());
//---close the reader and writer---
br.Close();
bw.Close();
This program first uses the File
class to open two files — one for reading and one for writing. The BinaryReader
class is then used to read the binary data from the FileStream
, and the BinaryWriter
is used to write the binary data to the file.
The BinaryReader
class contains many different read methods for reading different types of data — Read()
, Read7BitEncodedInt()
, ReadBoolean()
, ReadByte()
, ReadBytes()
, ReadChar()
, ReadChars()
, ReadDecimal()
, ReadDouble()
, ReadInt16()
, ReadInt32()
, ReadInt64()
, ReadSByte()
, ReadSingle()
, ReadString()
, ReadUInt16()
, ReadUInt32()
, and ReadUInt64()
.
- Shared Cache file
- Безопасность внешних таблиц. Параметр EXTERNAL FILE DIRECTORY
- Разработка приложений баз данных InterBase на Borland Delphi
- 4.4.4 The Dispatcher
- Open Source Insight and Discussion
- SERVER WORKING SIZE
- Introduction to Microprocessors and Microcontrollers
- About the author
- Chapter 6. Traversing of tables and chains
- Chapter 7. The state machine
- Chapter 8. Saving and restoring large rule-sets
- Chapter 11. Iptables targets and jumps